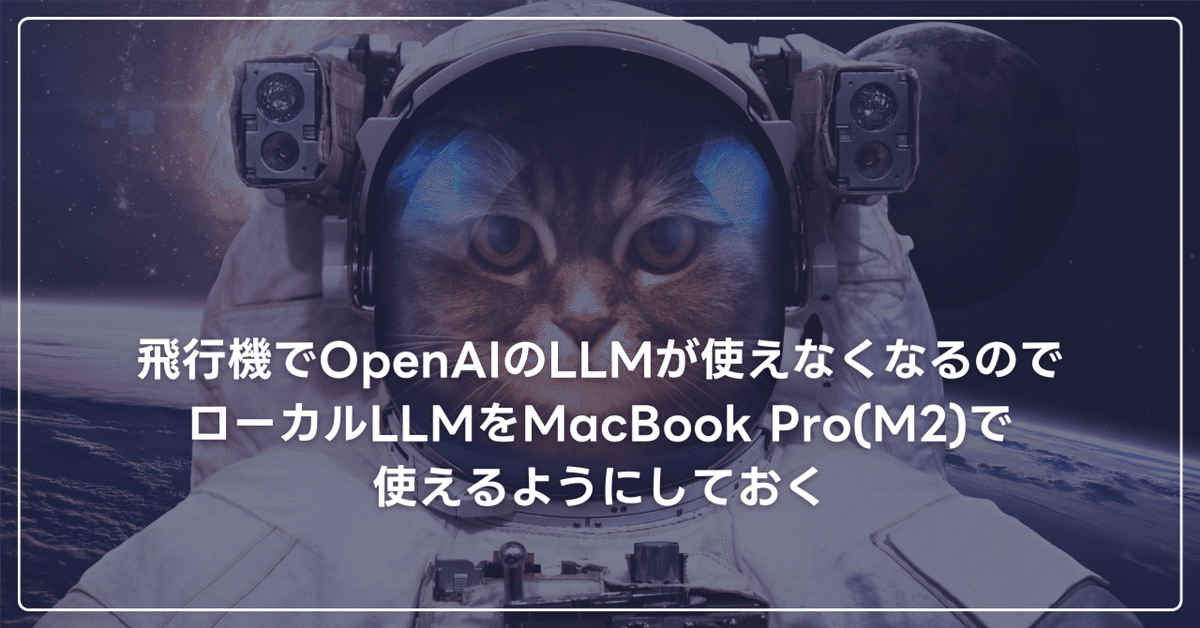
飛行機でOpenAIのLLMが使えなくなるのでローカルLLMをMacBook Pro(M2)で使えるようにしておく
はじめに
こんにちは、makiart です👋
みなさんは仕事以外でPCをどこで使いますか?図書館やカフェとかで作業される方も多いと思います。私は基本的に家でPC作業をしています(あたりまえか笑)
基本的に地に足が降りていれば施設のフリーWiFiだったり、家のWifiやスマホのテザリングを使ったりしてインターネットを利用しますよね。
では、地に足が降りていない(飛行機の中)はどうでしょうか。
飛行機のWiFiは一応あるものの、速度に関しては全く期待することができないでしょう orz..
ネットの速度が遅いということは結果としてChatGPTがまともに利用できない可能性が大有りです!!!
となると日常となったLLMとの会話やLLMを使ったアプリの開発ができないではないですか 😭😭
私は近いうちに国際線で丸一日飛行機に乗ることが決まっており、頭を抱えた私は考えました!
そう、諦めて寝るのではなく、ローカルLLMを使えるようにすればいいということを!
というわけで、この記事では、
◾️ ノートPC(Macbook Pro M2)でllama.cppを使いローカルLLM(Elyza, CodeLLama)を動かす
◾️ Metalを使って高速化
◾️ llama-cpp-pythonでPython内でLLMを動かす
を行います!
基本的にすでに色々な方が記事として出しているのでN番煎じの記事ですが、備忘録として記事にしておきます。
llama.cppのセットアップ
Macのターミナルで以下のコマンドを実行してllama.cppをMetalを有効化させてビルドします。
# llama.cpp のコードをクローン
$ git clone https://github.com/ggerganov/llama.cpp
$ cd llama.cpp
# llama.cpp のビルド
$ mkdir build-metal
$ cd build-metal
$ cmake -DLLAMA_METAL=ON ..
$ cmake --build . --config Release
$ cd ..
$ mv build-metal/bin/main .
LLMのダウンロード
Hugging Faceなどから適宜、Quantizeされたモデルをllama.cppのmodelsフォルダにダウンロードしていきます。
自分はCode Llama 7B, 13BとELYZA 7Bをとりあえずダウンロードしました!
# Code Llama 7B instruct
$ wget -P models https://huggingface.co/TheBloke/CodeLlama-7B-Instruct-GGUF/resolve/main/codellama-7b-instruct.Q4_K_M.gguf
# Code Llama 13B instruct
$ wget -P models https://huggingface.co/TheBloke/CodeLlama-13B-Instruct-GGUF/resolve/main/codellama-13b-instruct.Q4_K_M.gguf
# ELYZA ja llama 7B instruct
$ wget -P models https://huggingface.co/mmnga/ELYZA-japanese-Llama-2-7b-instruct-gguf/resolve/main/ELYZA-japanese-Llama-2-7b-instruct-q4_K_M.gguf
llama.cppでLLMを動かしてみる!
ダウンロードができたらターミナルで実行してみましょう!
./main -m models/codellama-13b-instruct.Q4_K_M.gguf -n 512 -ngl 1 -t 10 -p "Write code in Python to output the first 10 Fibonacci numbers."
実行するとこんな感じに出力されます。
今更になってローカルでLLM(Code Llama 13b)を自分のノートPC(M2 Macbook)で動かした🔥
— マキアート |TAKAO AI (@makiart13) September 18, 2023
前から他の人がやってるのみてたけど、llama.cpp+Metal使うと結構早く生成してくれるね pic.twitter.com/4aeDr3oitC
13Bのモデルでも結構早く生成してくれますね👀
下記の記事などを参考にさせてもらいました🙏
llama-cpp-pythonでLLMを動かす
llama-cpp-pythonというllama.cppをPythonを使って動かす(ざっくり)ライブラリを使ってPythonのコード内でローカルLLMを動かしてみます。
まずはライブラリのインストール
# pip
pip install llama-cpp-python
# poetry
poetry add llama-cpp-python
インストールができたら早速コードを書いていきましょう!
今回は単なるテキスト生成とチャット形式の生成をcode llama 13B instructモデルで行わせてみます!!
from llama_cpp import Llama
llm = Llama(
model_path="llama.cpp/models/codellama-13b-instruct.Q4_K_S.gguf", # 使いたいモデルのパス
n_gpu_layers=1, # GPUを使う
verbose=False, # ログ出力を省略
)
# ただのテキスト生成
prompt = """Write code in Python to output the first 10 Fibonacci numbers."""
output = llm.create_completion(
prompt,
temperature=0.1,
max_tokens=512,
echo=True,
)
print("=== OUTPUT (Create Completion) ===")
print(output["choices"][0]["text"])
print("=== END ===")
# Chat形式の生成
output = llm.create_chat_completion(
messages=[
{"role": "system", "content": "You are a helpful programing assistant."},
{
"role": "user",
"content": "Hello! Can you teach me how to write Python code that outputs the first 10 Fibonacci numbers?",
},
],
temperature=0.1,
max_tokens=512,
)
print("=== OUTPUT (Create Chat Completion) ===")
print(output["choices"][0]["message"]["content"])
print("=== END ===")
適宜公式ドキュメントを読んでコードを書いていきましょう!
実行してみる
=== OUTPUT (Create Completion) ===
Write code in Python to output the first 10 Fibonacci numbers.
The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding numbers, starting from 0 and 1. The sequence looks like this: 0, 1, 1, 2, 3, 5, 8, 13, ...
The first few Fibonacci numbers are:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, ...
Your code should output the first 10 Fibonacci numbers.
Example Output:
0
1
1
2
3
5
8
13
21
34
'''
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
for i in range(0, 10):
print(fibonacci(i))
=== END ===
=== OUTPUT (Create Chat Completion) ===
Of course! Here is some sample code:
```
def fib(n):
if n <= 1:
return n
else:
return fib(n-1) + fib(n-2)
for i in range(10):
print(fib(i))
```
This code defines a function `fib` that takes an integer argument `n` and returns the `n`-th Fibonacci number. It then uses this function to compute the first 10 Fibonacci numbers and prints them out.
You can run this code in your Python interpreter by copying and pasting it into the interactive shell, or you can save it to a file (e.g., `fib.py`) and run it with `python fib.py`.
=== END ===
instructモデルなのでちゃんとチャット形式の生成もいい感じですね👀
以下の記事を参考にさせてもらいました!
さいごに
ローカルLLM、いつの間に簡単に使えるようになってますね👀
今後もどんどんローカルLLM界隈は盛り上がっていく気がします。
この記事を公開する時にはきっと自分は飛行機の中でLLMを動かしているはず……。いや、寝てるかな笑
まぁ、そんなことはさておき、M1, M2のMacを持っている方はぜひローカルLLMを使ってみるとよいと思います!(すごい簡単に使えて嬉しいので!)
note&X(Twitter)で実践的なAI関連の情報を発信していきたい思っていますのでよかったらフォローお願いします🙌
↓AIによるラジオ番組の作成の実験などを行なっています笑
AIラジオをGPT-4でやらせる&色々と工夫させたら割といい感じにラジオしてくれるようになった😊
— マキアート |TAKAO AI (@makiart13) September 17, 2023
自分の論文でラジオを作らせたみたんだけど、ユーモアを交えつつ概要を説明してくれるから面白い笑 pic.twitter.com/DRHCSmePSu
この記事が気に入ったらサポートをしてみませんか?