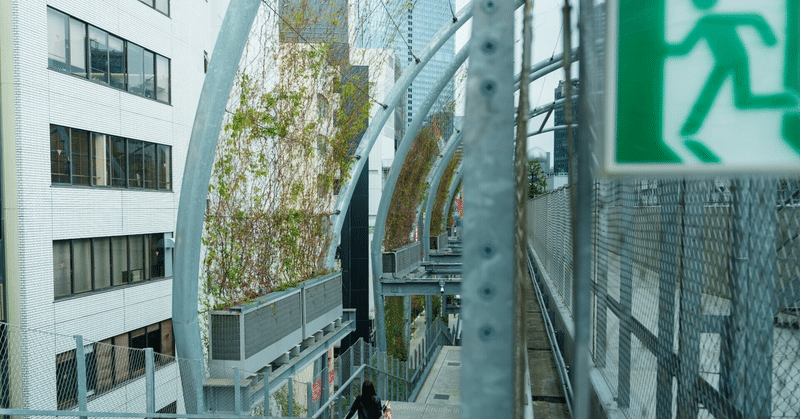
Photo by
wh_o_key
MT4に指標を表示するインジケーター ソースコード付き
前回の記事
指標全件と、直近24時間の切り替え機能をつけてあります。
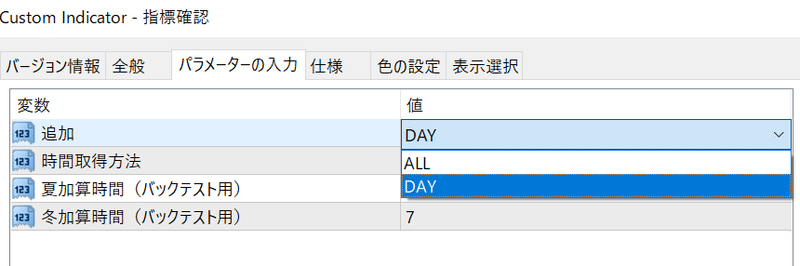
全件モード
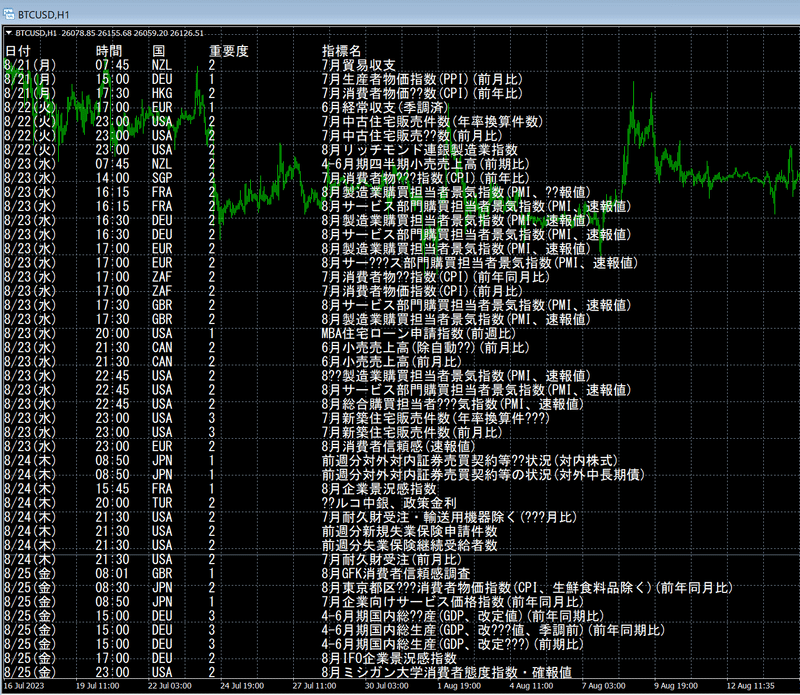
直近24時間モード
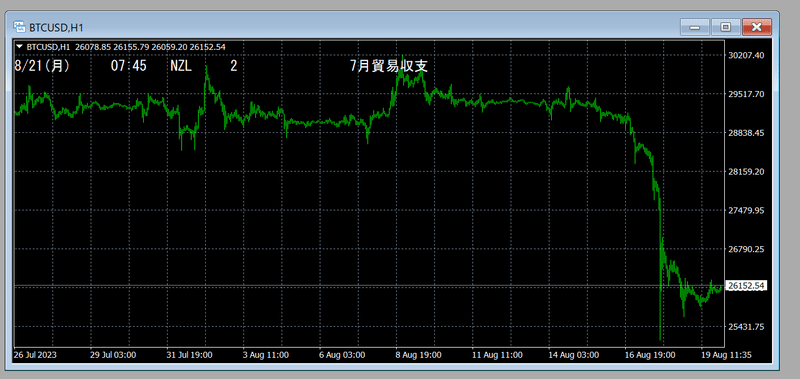
ダウンロード
ソースコード
//+------------------------------------------------------------------+
//| 指標確認.mq4 |
//| Copyright 2023, MetaQuotes Ltd. |
//| https://www.mql5.com |
//+------------------------------------------------------------------+
#property copyright "Copyright 2023, MetaQuotes Ltd."
#property link "https://www.mql5.com"
#property version "1.00"
#property strict
#property indicator_chart_window
enum all_or_day {ALL, DAY}; // 追加
input all_or_day displayOption = DAY; // 追加
#import "wininet.dll"
int InternetOpenW(string agent, int accessType, string proxyName, string proxyByPass, int flags);
int InternetConnectW(int internet, string serverName, int port, string userName, string password, int service, int flags, int context);
int HttpOpenRequestW(int connect, string verb, string objectName, string version, string referer, char& acceptTypes[], uint flags, int context);
bool HttpSendRequestW(int hRequest, string &lpszHeaders, int dwHeadersLength, uchar &lpOptional[], int dwOptionalLength);
int InternetReadFile(int, uchar &arr[], int, int &byte);
int InternetCloseHandle(int winINet);
#import
#define DEFAULT_HTTPS_PORT 443
#define SERVICE_HTTP 3
#define FLAG_SECURE 0x00800000
#define FLAG_PRAGMA_NOCACHE 0x00000100
#define FLAG_KEEP_CONNECTION 0x00400000
#define FLAG_RELOAD 0x80000000
string your_url = "us-central1-optical-legend-324601.cloudfunctions.net";
string your_endpoint = "/sihyou";
#import "kernel32.dll"
int MultiByteToWideChar(uint CodePage, uint dwFlags, string lpMultiByteStr, int cbMultiByte, string lpWideCharStr, int cchWideChar);
#import
#define CP_UTF8 65001
string GetDataFromServer() {
string host = your_url;
string UserAgent = "MT4 Indicator";
string nill = "";
int session = InternetOpenW(UserAgent, 0, nill, nill, 0);
int connect = InternetConnectW(session, host, DEFAULT_HTTPS_PORT, nill, nill, SERVICE_HTTP, 0, 0);
string Vers = "HTTP/1.1";
string Method = "GET";
string Object = your_endpoint;
string Types = "";
char acceptTypes[];
StringToCharArray(Types, acceptTypes);
int context = 0;
int hRequest = HttpOpenRequestW(connect, Method, Object, Vers, nill, acceptTypes, FLAG_SECURE | FLAG_KEEP_CONNECTION | FLAG_RELOAD | FLAG_PRAGMA_NOCACHE, context);
string headers = "Content-Type: application/x-www-form-urlencoded\r\n";
uchar body[];
int dwHeadersLength = 0;
int dwOptionalLength = 0;
bool hSend = HttpSendRequestW(hRequest, headers, dwHeadersLength, body, dwOptionalLength);
uchar ch[100];
string toStr = "";
int dwBytes;
while(InternetReadFile(hRequest, ch, 100, dwBytes)) {
if(dwBytes <= 0) break;
toStr += CharArrayToString(ch, 0, dwBytes,CP_UTF8);
}
InternetCloseHandle(hSend);
InternetCloseHandle(hRequest);
InternetCloseHandle(connect);
InternetCloseHandle(session);
return toStr;
}
// テキストオブジェクトを作成する関数
void CreateObject(string name, string text, int row, int col) {
ObjectCreate(0, name, OBJ_LABEL, 0, 0, 0);
ObjectSetInteger(0, name, OBJPROP_XDISTANCE, col);
ObjectSetInteger(0, name, OBJPROP_YDISTANCE, 30 + row * 25);
ObjectSetText(name, text, 12, "MS Gothic", clrWhite);
}
// CSVデータを解析する関数
void ParseCSVData(string csvData) {
int start = 0;
int end = 0;
int row = 0;
datetime currentTime = getJapanTime();
while (start < StringLen(csvData)) {
end = StringFind(csvData, "\n", start);
if (end == -1) end = StringLen(csvData); // 最後の行の場合
string line = StringSubstr(csvData, start, end - start);
string date, time, country, eventName, importance;
date = StringSubstr(line, 0, StringFind(line, ","));
line = StringSubstr(line, StringFind(line, ",") + 1);
time = StringSubstr(line, 0, StringFind(line, ","));
line = StringSubstr(line, StringFind(line, ",") + 1);
country = StringSubstr(line, 0, StringFind(line, ",") );
line = StringSubstr(line, StringFind(line, ",") + 1);
eventName = StringSubstr(line, 0, StringFind(line, ","));
line = StringSubstr(line, StringFind(line, ",") + 1);
importance = StringSubstr(line, StringFind(line, ",") + 1);
// DAYオプションが選択された場合、現在の時間の前後24時間のデータのみを表示
if (displayOption == DAY) {
datetime currentYear = TimeYear(TimeCurrent());
string yearStr = IntegerToString(currentYear);
string month = StringSubstr(date, 0, StringFind(date, "/"));
string day = StringSubstr(date, StringFind(date, "/") + 1, StringFind(date, "(") - StringFind(date, "/") - 1);
datetime eventTime = StrToTime(yearStr + "." + month + "." + day + " " + time);
if (MathAbs(currentTime - eventTime) > 24 * 60 * 60) {
start = end + 1;
continue;
}
}
CreateObject("Date" + IntegerToString(row), date, row, 0);
CreateObject("Time" + IntegerToString(row), time, row, 150);
CreateObject("Country" + IntegerToString(row), country, row, 250);
CreateObject("EventName" + IntegerToString(row), eventName, row, 350);
CreateObject("Importance" + IntegerToString(row), importance, row, 550);
start = end + 1;
row++;
}
}
int OnInit() {
string data = GetDataFromServer();
Print("Received data from server: ", data);
ParseCSVData(data);
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Custom indicator iteration function |
//+------------------------------------------------------------------+
int OnCalculate(const int rates_total,
const int prev_calculated,
const datetime &time[],
const double &open[],
const double &high[],
const double &low[],
const double &close[],
const long &tick_volume[],
const long &volume[],
const int &spread[])
{
//---
//--- return value of prev_calculated for next call
return(rates_total);
}
//+------------------------------------------------------------------+
enum use_times {
GMT9, //WindowsPCの時間を使って計算する
GMT9_BACKTEST, // EAで計算された時間を使う(バックテスト用)
GMT_KOTEI// サーバータイムがGMT+0で固定されている(バックテスト用)
};
input use_times set_time = GMT9;//時間取得方法
input int natu = 6;//夏加算時間(バックテスト用)
input int huyu = 7;//冬加算時間(バックテスト用)
datetime calculate_time() {
if(set_time == GMT9) {
return TimeGMT() + 60 * 60 * 9;
}
if(set_time == GMT9_BACKTEST) {
return getJapanTime();
}
if(set_time == GMT_KOTEI) {
return TimeCurrent() + 60 * 60 * 9;
}
return 0;
}
// 日本時間の取得
datetime getJapanTime() {
datetime now = TimeCurrent();
datetime summer = now + 60 * 60 * natu;
datetime winter = now + 60 * 60 * huyu;
if(is_summer()) {
return summer;
}
return winter;
}
// サマータイムなら真を返す関数
bool is_summer() {
datetime now = TimeCurrent();
int year = TimeYear(now);
int month = TimeMonth(now);
int day = TimeDay(now);
int dayOfWeek = TimeDayOfWeek(now);
int hours = TimeHour(now);
if (month < 3 || month > 11) {
return false;
}
if (month > 3 && month < 11) {
return true;
}
// アメリカのサマータイムは3月の第2日曜日から11月の第1日曜日まで
if (month == 3) {
int dstStart = 14 - dayOfWeek;
if (day >= dstStart) {
return true;
} else {
return false;
}
}
if (month == 11) {
int dstEnd = 7 - dayOfWeek;
if (day < dstEnd) {
return true;
} else {
return false;
}
}
return false;
}
ここから先は
0字
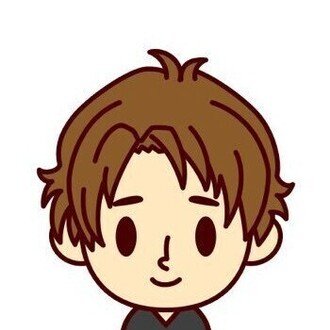
このマガジンで読み放題です。
FX自動売買開発入門サンプルコードセット
10,000円
EA開発者のためのサンプルコード集
この記事が気に入ったらサポートをしてみませんか?