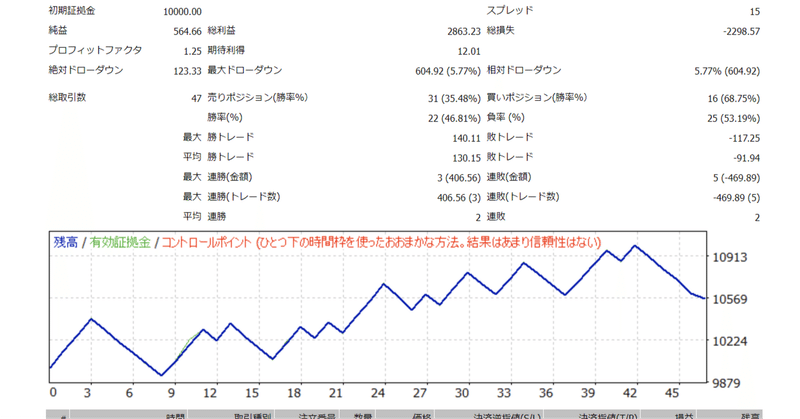
ChatGPTでEMAクロスのEAを作成する
プロンプト
以下の条件でMQL4を使ってMT4のEAを作成してください。
・EMA10.EMA20のクロス
・SMA20の位置より上ならbuy・下ならsell
・bb20偏差0.1のラインより上ならbuy・下ならsell
条件です。
利確・損切りは適当です。
MagicNumberは321
以下の手順でプログラムを組み立ててください。
void position_entry(int side) をつくる
void position_close(int side) をつくる
int position_count(int side) をつくる
bool is_buy() をつくる
シグナルは
position_count(0)が0
条件ABC
A EMAのゴールデンクロス、デッドクロス
B SMAと終値の比較
C BB偏差値1.0と終値の比較
BUYエントリー条件
A EMAのゴールデンクロス
B SMA < 終値
C BB < 終値
SELLエントリー条件
BUYの逆
注意点として
OnTick()内部で各関数を呼び出すこと
position_countはMagicNumberを含めること
説明コメントは不要です
ストップロス、テイクプロフィットは不要です。
バックテスト
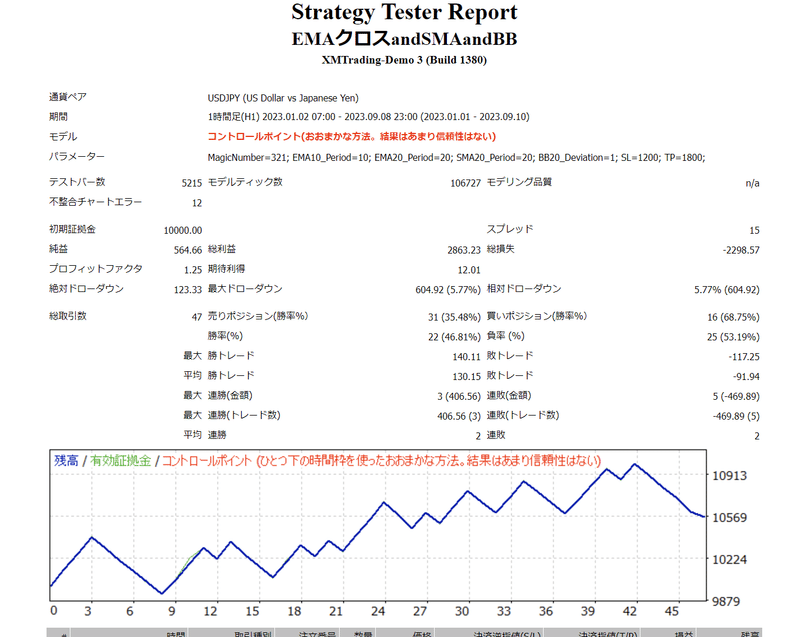
ソースコード
//+------------------------------------------------------------------+
//| Expert Advisor - カスタムEA |
//| Copyright 2023, Your Name Here |
//| https://www.yourwebsite.com |
//+------------------------------------------------------------------+
#property strict
input int MagicNumber = 321;
input int EMA10_Period = 10;
input int EMA20_Period = 20;
input int SMA20_Period = 20;
input double BB20_Deviation = 1.0;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
// 初期化コードをここに記述
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
// デイニシャライズコードをここに記述
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
if (position_count(0) == 0 && position_count(1) == 0)
{
if (is_buy())
{
position_entry(OP_BUY);
}
else if (is_sell())
{
position_entry(OP_SELL);
}
}
}
input int SL = 1200;
input int TP = 1800;
void position_entry(int side)
{
double qty = 0.1;
double entryPrice = 0.0;
double slPrice = 0.0;
double tpPrice = 0.0;
if (side == 0)
{
entryPrice = Ask;
slPrice = entryPrice - SL * Point; // SLはエントリー価格から200 * Point下に設定
tpPrice = entryPrice + TP * Point; // TPはエントリー価格から200 * Point上に設定
int ticket = OrderSend(NULL, side, qty, entryPrice, 0, slPrice, tpPrice, NULL, MagicNumber, 0, clrGreen);
if (ticket > 0)
{
Print("Long Order Placed. Ticket: ", ticket);
}
else
{
Print("Error placing Long Order. Error code: ", GetLastError());
}
}
else if (side == 1)
{
entryPrice = Bid;
slPrice = entryPrice + SL * Point; // SLはエントリー価格から200 * Point上に設定
tpPrice = entryPrice - TP * Point; // TPはエントリー価格から200 * Point下に設定
int ticket = OrderSend(NULL, side, qty, entryPrice, 0, slPrice, tpPrice, NULL, MagicNumber, 0, clrRed);
if (ticket > 0)
{
Print("Short Order Placed. Ticket: ", ticket);
}
else
{
Print("Error placing Short Order. Error code: ", GetLastError());
}
}
}
int position_count(int side)
{
int count = 0;
for (int i = 0; i < OrdersTotal(); i++)
{
if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES))
{
if (OrderMagicNumber() == MagicNumber)
{
if (side == 0 || OrderType() == side)
{
count++;
}
}
}
}
return count;
}
bool is_buy()
{
double ema10_shift1 = iMA(Symbol(), 0, EMA10_Period, 0, MODE_EMA, PRICE_CLOSE, 1);
double ema20_shift1 = iMA(Symbol(), 0, EMA20_Period, 0, MODE_EMA, PRICE_CLOSE, 1);
double ema10_shift2 = iMA(Symbol(), 0, EMA10_Period, 0, MODE_EMA, PRICE_CLOSE, 2);
double ema20_shift2 = iMA(Symbol(), 0, EMA20_Period, 0, MODE_EMA, PRICE_CLOSE, 2);
double sma20_shift1 = iMA(Symbol(), 0, SMA20_Period, 0, MODE_SMA, PRICE_CLOSE, 1);
double bb20_shift1 = iBands(Symbol(), 0, 20, BB20_Deviation, 0, PRICE_CLOSE, MODE_MAIN, 1);
if (ema10_shift1 > ema20_shift1 && ema10_shift2 <= ema20_shift2 && sma20_shift1 < Close[1] && bb20_shift1 < Close[1])
{
return true;
}
return false;
}
bool is_sell()
{
double ema10_shift1 = iMA(Symbol(), 0, EMA10_Period, 0, MODE_EMA, PRICE_CLOSE, 1);
double ema20_shift1 = iMA(Symbol(), 0, EMA20_Period, 0, MODE_EMA, PRICE_CLOSE, 1);
double ema10_shift2 = iMA(Symbol(), 0, EMA10_Period, 0, MODE_EMA, PRICE_CLOSE, 2);
double ema20_shift2 = iMA(Symbol(), 0, EMA20_Period, 0, MODE_EMA, PRICE_CLOSE, 2);
double sma20_shift1 = iMA(Symbol(), 0, SMA20_Period, 0, MODE_SMA, PRICE_CLOSE, 1);
double bb20_shift1 = iBands(Symbol(), 0, 20, BB20_Deviation, 0, PRICE_CLOSE, MODE_MAIN, 1);
if (ema10_shift1 < ema20_shift1 && ema10_shift2 >= ema20_shift2 && sma20_shift1 > Close[1] && bb20_shift1 > Close[1])
{
return true;
}
return false;
}
この記事が気に入ったらサポートをしてみませんか?