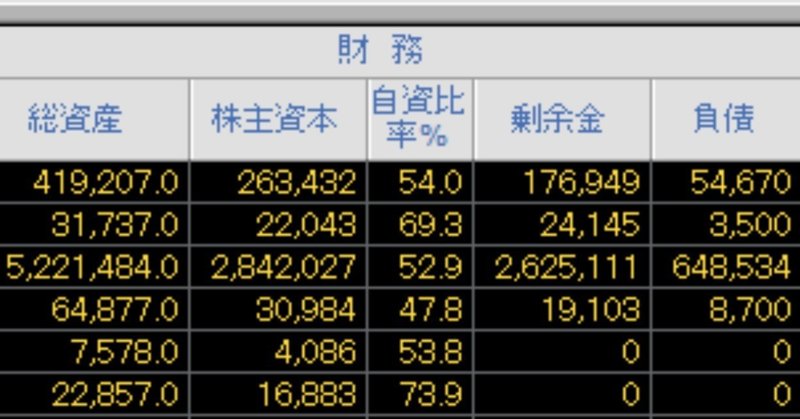
財務
既存のインジケータまとめて使ってるよシリーズ第4弾は財務です
必要そうなものから紹介していってるので段々しょぼくなるのは仕様です(・∀・)
任意の新しいインジケータを作成します
既存のインジケータの総資産・株主資本、自己資本比率に、
剰余金と負債を入力できる項目を追加しています
殆ど変わらない項目なので、少数のPF管理用でデータは手入力です
剰余金、負債を入力しない場合は初期値の0が表示されます
多くの銘柄を管理したい場合は、外部からデータを用意してCSV等で!
プログラム
using tsdata.marketdata;
using elsystem;
inputs:
int MonthsReported( 12 ) [DisplayName = "期間", ToolTip =
"Enter the number of months in the reporting period for which data is desired. Fundamental data usually covers a 3, 6 or 12 month period."],
int ConsolidationLevel( 1 ) [DisplayName = "連結/単独", ToolTip =
"Enter 1 if consolidated data is desired; enter any other value for non-consolidated data."],
int AccountingStandard( 0 ) [DisplayName = "会計基準", ToolTip =
"Enter 1 for Japanese accounting standard; enter 2 for Securities and Exchange Commission (SEC) accounting standard; enter 3 for International Financial Reporting Standards (IFRS) accounting standard; enter 0 to use IFRS, SEC, or Japanese accounting standards, in that order, depending on which is available."],
PlotTotalAssets( 1 ) [DisplayName = "総資産をプロット", ToolTip =
"Enter 1 if it is desired to plot total assets; enter a value other than 1 if it is not desired to plot this value."],
PlotNetAssets( 1 ) [DisplayName = "株主資本をプロット", ToolTip =
"Enter 1 if it is desired to plot net assets; enter a value other than 1 if it is not desired to plot this value."],
int Rieki( 0 )[DisplayName = "利益剰余金", ToolTip = "利益剰余金"],
int Husai( 0 )[DisplayName = "有利子負債", ToolTip = "有利子負債"];
variables:
FundamentalQuotesProvider FQP( null ),
intrabarpersist bool OkayToPlotTotalAssets( false ),
intrabarpersist bool OkayToPlotNetAssets( false ),
intrabarpersist bool OkayToPlotRatio( false ),
intrabarpersist string ConsLevel( "Consolidated" ),
intrabarpersist string AcctStdInputString( "" ),
double CRTotalAssets( 0 ),
double CRNetAssets( 0 ),
double CRCapitalRatio( 0 );
constants:
string MonthsReportedKey( "MonthsReported" ),
string ConsolidatedKey( "ConsolidationLevel" ),
string AccountingKey( "AccountingStandard" ),
string TotalAssetsFieldName( "CR_Total_Asset" ),
string NetAssetsFieldName( "CR_Net_Asset" ),
string RatioFieldName( "CR_Capital_Ratio" ),
string ConsolidatedValue( "Consolidated" ),
string NonConsolidatedValue( "NonConsolidated" ),
string IFRSString( "IFRS" ),
string SECString( "SEC" ),
string JSTDString( "JSTD" );
method void CreateFundamentalQuotesProvider()
begin
FQP = new FundamentalQuotesProvider();
FQP.Symbol = Symbol;
FQP.Fields += TotalAssetsFieldName;
FQP.Fields += NetAssetsFieldName;
FQP.Fields += RatioFieldName;
FQP.Realtime = false;
FQP.TimeZone = tsdata.common.TimeZone.local;
FQP.LoadProvider();
Value99 = FQP.Count; { force provider to load }
end;
method bool DataHasPostDate( FundamentalQuote fq )
variables: int Counter, DateTime MissingDT, int NumMissingDates;
begin
NumMissingDates = 0;
MissingDT = DateTime.Create( 1999, 11, 30 );
for Counter = 0 to fq.Count - 1 begin
if fq.PostDate[Counter] = MissingDT then
NumMissingDates += 1;
end;
return NumMissingDates <> fq.Count;
end;
method string GetAcctStdString()
variables: string AcctStdString;
begin
switch ( AccountingStandard )
begin
case 1: { JSTD }
AcctStdString = JSTDString;
case 2: { SEC }
AcctStdString = SECString;
case 3: { IFRS }
AcctStdString = IFRSString;
end;
return AcctStdString;
end;
method bool GetQuoteAsOfDate( FundamentalQuote fq, DateTime tempdt,
out double QuoteVal )
variables:
int Counter,
bool QuoteFound,
DateTime LastDateTime,
string AcctStd,
string LastUsedAcctStd,
bool SetVariable,
DateTime fqDateTime;
begin
if fq = NULL or tempdt = NULL then return false;
QuoteFound = false;
LastDateTime = DateTime.Create( 1900, 1, 1 );
SetVariable = false;
for Counter = fq.Count - 1 downto 0 begin
SetVariable = false;
AcctStd = fq.ExtendedProperties[Counter][AccountingKey].StringValue;
if fq.ExtendedProperties[Counter][MonthsReportedKey].IntegerValue = MonthsReported
and fq.ExtendedProperties[Counter][ConsolidatedKey].StringValue = ConsLevel
and ( AccountingStandard = 0 or AcctStd = AcctStdInputString ) then begin
fqDateTime = fq.PostDate[Counter];
if tempdt >= fqDateTime and fqDateTime >= LastDateTime then begin
if fqDateTime <> LastDateTime then begin { new PostDate }
SetVariable = true;
end else if AccountingStandard = 0 then begin { use hierarchy }
if AcctStd = IFRSString then
begin
SetVariable = true;
end else if AcctStd = SECString and LastUsedAcctStd <> IFRSString then begin
SetVariable = true;
end else if AcctStd = JSTDString
and LastUsedAcctStd <> IFRSString
and LastUsedAcctStd <> SECString then begin
SetVariable = true;
end;
end;
end;
end;
if SetVariable then begin
QuoteVal = fq.DoubleValue[Counter];
LastUsedAcctStd = AcctStd;
LastDateTime = fqDateTime;
QuoteFound = true;
end;
end;
return QuoteFound;
end;{ GetQuoteAsOfDate method }
once begin
if ConsolidationLevel = 1 then ConsLevel = ConsolidatedValue
else ConsLevel = NonConsolidatedValue;
AcctStdInputString = GetAcctStdString();
CreateFundamentalQuotesProvider();
OkayToPlotTotalAssets = FQP.HasQuoteData( TotalAssetsFieldName )
and DataHasPostDate( FQP[TotalAssetsFieldName] )
and PlotTotalAssets = 1;
OkayToPlotNetAssets = FQP.HasQuoteData( NetAssetsFieldName )
and DataHasPostDate( FQP[NetAssetsFieldName] )
and PlotNetAssets = 1;
OkayToPlotRatio = FQP.HasQuoteData( RatioFieldName )
and DataHasPostDate( FQP[RatioFieldName] );
end;
if OkayToPlotTotalAssets and GetQuoteAsOfDate( FQP[TotalAssetsFieldName], BarDateTime, CRTotalAssets ) then
Plot1( CRTotalAssets, !( "総資産" ) );
if OkayToPlotNetAssets and GetQuoteAsOfDate( FQP[NetAssetsFieldName], BarDateTime, CRNetAssets ) then
Plot2( CRNetAssets, !( "株主資本" ) );
if OkayToPlotRatio and GetQuoteAsOfDate( FQP[RatioFieldName], BarDateTime, CRCapitalRatio ) then
Plot3( CRCapitalRatio, !( "自資比率%" ) );
Plot4( Rieki, !( "剰余金" ) );
Plot5( Husai, !( "負債" ) );
サポートされると喜んでアイスを買っちゃいます!٩(๑❛ᴗ❛๑)۶