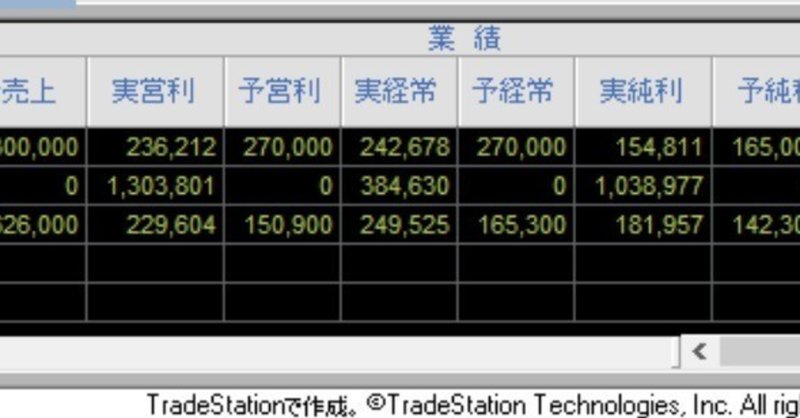
業績
既存のインジケータまとめて使ってるよシリーズ第3弾
業績に関するインジケータをまとめたものです
アプリの業績推移の元になったインジケータです
アプリを作る前は遡りの数を変えて過去の業績を見ていましたw
任意の新しいインジケータを作成します
既存のインジケータの売上高、営業利益、経常利益、純利益、営業利益率(%)、営業利益成長率をまとめて1つにして表示しているだけです
既存の物と見比べてインジケータの幅はほとんど変わりませんが、
レーダースクリーンに表示出来るインジケータ数はMAX30なので
既存の売上高、営業利益、経常利益、純利益、営業利益率(%)、営業利益成長率、と6つ使うより少なく
使用個数に余裕ができ、連結/単独の入力も1回で済みます
必要のない項目は見出し部分を右クリックしてプロットを表示/非表示で!
プログラム
using tsdata.marketdata;
using elsystem;
inputs:
int MonthsReported( 12 ) [DisplayName = "MonthsReported", ToolTip =
"Enter the number of months in the reporting period for which data is desired. Fundamental data usually covers a 3, 6 or 12 month period."],
int ConsolidationLevel( 1 ) [DisplayName = "ConsolidationLevel", ToolTip =
"Enter 1 if consolidated data is desired; enter any other value for non-consolidated data."],
int AccountingStandard( 0 ) [DisplayName = "AccountingStandard", ToolTip =
"Enter 1 for Japanese accounting standard; enter 2 for Securities and Exchange Commission (SEC) accounting standard; enter 3 for International Financial Reporting Standards (IFRS) accounting standard; enter 0 to use IFRS, SEC, or Japanese accounting standards, in that order, depending on which is available."];
variables:
FundamentalQuotesProvider FQP( NULL ),
intrabarpersist bool OkayToPlot( false ),
intrabarpersist bool OkayToPlotActual( false ),
intrabarpersist bool OkayToPlotForecast( false ),
intrabarpersist string ConsLevel( "Consolidated" ),
intrabarpersist string AcctStdInputString( "" ),
double CR_Sales( 0 ),
double CE_Sales( 0 ),
double CROp( 0 ),
double CEOp( 0 ),
double CRRP( 0 ),
double CERP( 0 ),
double CRNP( 0 ),
double CENP( 0 ),
double CROPSalesRatio( 0 ),
double CROpYoY( 0 ),
double CEOpYoY( 0 );
constants:
string MonthsReportedKey( "MonthsReported" ),
string ConsolidatedKey( "ConsolidationLevel" ),
string AccountingKey( "AccountingStandard" ),
string SALESActualFieldName( "CR_SALES" ),
string SALESForecastFieldName( "CE_SALES" ),
string OPActualFieldName( "CR_Op" ),
string OPForecastFieldName( "CE_Op" ),
string RPActualFieldName( "CR_RP" ),
string RPForecastFieldName( "CE_RP" ),
string NPActualFieldName( "CR_NP" ),
string NPForecastFieldName( "CE_NP" ),
string OperatingProfitFieldName( "CR_OP_Sales_Ratio" ),
string OPYOYActualFieldName( "CR_Op_YoY" ),
string OPYOYForecastFieldName( "CE_Op_YoY" ),
string ConsolidatedValue( "Consolidated" ),
string NonConsolidatedValue( "NonConsolidated" ),
string IFRSString( "IFRS" ),
string SECString( "SEC" ),
string JSTDString( "JSTD" );
method void CreateFundamentalQuotesProvider()
begin
FQP = new FundamentalQuotesProvider();
FQP.Symbol = Symbol;
FQP.Fields += SALESActualFieldName;
FQP.Fields += SALESForecastFieldName;
FQP.Fields += OPActualFieldName;
FQP.Fields += OPForecastFieldName;
FQP.Fields += RPActualFieldName;
FQP.Fields += RPForecastFieldName;
FQP.Fields += NPActualFieldName;
FQP.Fields += NPForecastFieldName;
FQP.Fields += OperatingProfitFieldName;
FQP.Fields += OPYOYActualFieldName;
FQP.Fields += OPYOYForecastFieldName;
FQP.Realtime = false;
FQP.TimeZone = tsdata.common.TimeZone.local;
FQP.LoadProvider();
Value99 = FQP.Count; { force provider to load }
end;
method bool DataHasPostDate( FundamentalQuote fq )
variables: int Counter, DateTime MissingDT, int NumMissingDates;
begin
NumMissingDates = 0;
MissingDT = DateTime.Create( 1999, 11, 30 );
for Counter = 0 to fq.Count - 1
begin
if fq.PostDate[Counter] = MissingDT then
NumMissingDates += 1;
end;
return NumMissingDates <> fq.Count;
end;
method string GetAcctStdString()
variables: string AcctStdString;
begin
switch ( AccountingStandard )
begin
case 1: { JSTD }
AcctStdString = JSTDString;
case 2: { SEC }
AcctStdString = SECString;
case 3: { IFRS }
AcctStdString = IFRSString;
end;
return AcctStdString;
end;
method bool GetQuoteAsOfDate( FundamentalQuote fq, DateTime tempdt,
out double QuoteVal )
variables:
int Counter,
bool QuoteFound,
DateTime LastDateTime,
string AcctStd,
string LastUsedAcctStd,
bool SetVariable,
DateTime fqDateTime;
begin
if fq = NULL or tempdt = NULL then return false;
QuoteFound = false;
LastDateTime = DateTime.Create( 1900, 1, 1 );
SetVariable = false;
for Counter = fq.Count - 1 downto 0 begin
SetVariable = false;
AcctStd = fq.ExtendedProperties[Counter][AccountingKey].StringValue;
if fq.ExtendedProperties[Counter][MonthsReportedKey].IntegerValue = MonthsReported
and fq.ExtendedProperties[Counter][ConsolidatedKey].StringValue = ConsLevel
and ( AccountingStandard = 0 or AcctStd = AcctStdInputString ) then begin
fqDateTime = fq.PostDate[Counter];
if tempdt >= fqDateTime and fqDateTime >= LastDateTime then begin
if fqDateTime <> LastDateTime then begin { new PostDate }
SetVariable = true;
end else if AccountingStandard = 0 then begin { use hierarchy }
if AcctStd = IFRSString then begin
SetVariable = true;
end else if AcctStd = SECString and LastUsedAcctStd <> IFRSString then begin
SetVariable = true;
end else if AcctStd = JSTDString
and LastUsedAcctStd <> IFRSString
and LastUsedAcctStd <> SECString then begin
SetVariable = true;
end;
end;
end;
end;
if SetVariable then begin
QuoteVal = fq.DoubleValue[Counter];
LastUsedAcctStd = AcctStd;
LastDateTime = fqDateTime;
QuoteFound = true;
end;
end;
return QuoteFound;
end;{ GetQuoteAsOfDate method }
once begin
if ConsolidationLevel = 1 then
ConsLevel = ConsolidatedValue
else
ConsLevel = NonConsolidatedValue;
AcctStdInputString = GetAcctStdString();
CreateFundamentalQuotesProvider();
OkayToPlotActual = FQP.HasQuoteData( SALESActualFieldName )
and DataHasPostDate( FQP[SALESActualFieldName] );
OkayToPlotForecast = FQP.HasQuoteData( SALESForecastFieldName )
and DataHasPostDate( FQP[SALESForecastFieldName] );
OkayToPlotActual = FQP.HasQuoteData( OPActualFieldName )
and DataHasPostDate( FQP[OPActualFieldName] );
OkayToPlotForecast = FQP.HasQuoteData( OPForecastFieldName )
and DataHasPostDate( FQP[OPForecastFieldName] );
OkayToPlotActual = FQP.HasQuoteData( RPActualFieldName )
and DataHasPostDate( FQP[RPActualFieldName] );
OkayToPlotForecast = FQP.HasQuoteData( RPForecastFieldName )
and DataHasPostDate( FQP[RPForecastFieldName] );
OkayToPlotActual = FQP.HasQuoteData( NPActualFieldName )
and DataHasPostDate( FQP[NPActualFieldName] );
OkayToPlotForecast = FQP.HasQuoteData( NPForecastFieldName )
and DataHasPostDate( FQP[NPForecastFieldName] );
OkayToPlot = FQP.HasQuoteData( OperatingProfitFieldName )
and DataHasPostDate( FQP[OperatingProfitFieldName] );
OkayToPlotActual = FQP.HasQuoteData( OPYOYActualFieldName )
and DataHasPostDate( FQP[OPYOYActualFieldName] );
OkayToPlotForecast = FQP.HasQuoteData( OPYOYForecastFieldName )
and DataHasPostDate( FQP[OPYOYForecastFieldName] );
end;
if OkayToPlotActual and GetQuoteAsOfDate( FQP[SALESActualFieldName], BarDateTime, CR_Sales ) then
Plot1( CR_Sales, !( "実売上" ) );
if OkayToPlotForecast and GetQuoteAsOfDate( FQP[SALESForecastFieldName], BarDateTime, CE_Sales ) then
Plot2( CE_Sales, !( "予売上" ) );
if OkayToPlotActual and GetQuoteAsOfDate( FQP[OPActualFieldName], BarDateTime, CROp ) then
Plot3( CROp, !( "実営利" ) );
if OkayToPlotForecast and GetQuoteAsOfDate( FQP[OPForecastFieldName], BarDateTime, CEOp ) then
Plot4( CEOp, !( "予営利" ) );
if OkayToPlotActual and GetQuoteAsOfDate( FQP[RPActualFieldName], BarDateTime, CRRP ) then
Plot5( CRRP, !( "実経常" ) );
if OkayToPlotForecast and GetQuoteAsOfDate( FQP[RPForecastFieldName], BarDateTime, CERP ) then
Plot6( CERP, !( "予経常" ) );
if OkayToPlotActual and GetQuoteAsOfDate( FQP[NPActualFieldName], BarDateTime, CRNP ) then
Plot7( CRNP, !( "実純利" ) );
if OkayToPlotForecast and GetQuoteAsOfDate( FQP[NPForecastFieldName], BarDateTime, CENP ) then
Plot8( CENP, !( "予純利" ) );
if OkayToPlot and GetQuoteAsOfDate( FQP[OperatingProfitFieldName], BarDateTime, CROPSalesRatio ) then
Plot9( CROPSalesRatio, !( "営利率%" ) );
if OkayToPlotActual and GetQuoteAsOfDate( FQP[OPYOYActualFieldName], BarDateTime, CROpYoY ) then
Plot10( CROpYoY, !( "実営利成率%" ) );
if OkayToPlotForecast and GetQuoteAsOfDate( FQP[OPYOYForecastFieldName], BarDateTime, CEOpYoY ) then
Plot11( CEOpYoY, !( "予営利成率%" ) );
サポートされると喜んでアイスを買っちゃいます!٩(๑❛ᴗ❛๑)۶