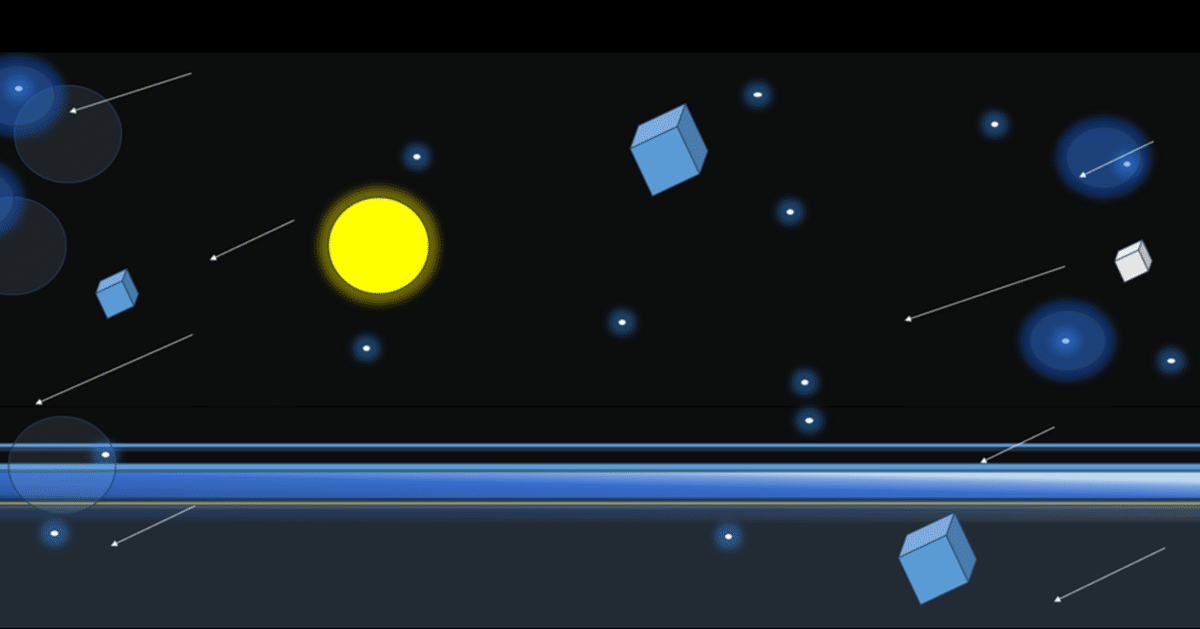
memo1127
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fuzzy Search Excel Table</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/xlsx/0.17.0/xlsx.full.min.js"></script>
</head>
<body>
<input type="file" id="excelFile" accept=".xlsx, .xls" />
<input type="text" id="searchInput" placeholder="Search..." />
<div id="output"></div>
<script>
document.getElementById('excelFile').addEventListener('change', handleFile);
document.getElementById('searchInput').addEventListener('input', handleSearch);
let originalData = [];
function handleFile(e) {
const file = e.target.files[0];
if (file) {
const reader = new FileReader();
reader.onload = function (e) {
const data = new Uint8Array(e.target.result);
const workbook = XLSX.read(data, { type: 'array' });
const sheetName = workbook.SheetNames[0];
const sheet = workbook.Sheets[sheetName];
originalData = XLSX.utils.sheet_to_json(sheet, { header: 1 });
displayData(originalData);
};
reader.readAsArrayBuffer(file);
}
}
function handleSearch() {
const searchTerm = document.getElementById('searchInput').value.toLowerCase();
const matchingRows = originalData.filter(row => row.some(cell => cell.toString().toLowerCase().includes(searchTerm)));
displayData(matchingRows);
}
function displayData(data) {
const outputDiv = document.getElementById('output');
outputDiv.innerHTML = '';
const table = document.createElement('table');
// ヘッダーの作成
const headerRow = document.createElement('tr');
for (let cell of data[0]) {
const th = document.createElement('th');
th.textContent = cell;
headerRow.appendChild(th);
}
table.appendChild(headerRow);
// データの表示
for (let i = 1; i < data.length; i++) {
const row = document.createElement('tr');
// 行を非表示にするスタイルを追加
row.style.display = 'none';
for (let cell of data[i]) {
const td = document.createElement('td');
td.textContent = cell;
row.appendChild(td);
}
table.appendChild(row);
}
outputDiv.appendChild(table);
showFuzzyMatchingRows(data);
}
function showFuzzyMatchingRows(data) {
const searchTerm = document.getElementById('searchInput').value.toLowerCase();
for (let i = 1; i < data.length; i++) {
const row = table.rows[i];
const cells = Array.from(row.cells);
if (cells.some(cell => cell.textContent.toLowerCase().includes(searchTerm))) {
// 検索結果に一致する行のみ表示する
row.style.display = '';
}
}
}
</script>
</body>
</html>
この記事が気に入ったらサポートをしてみませんか?