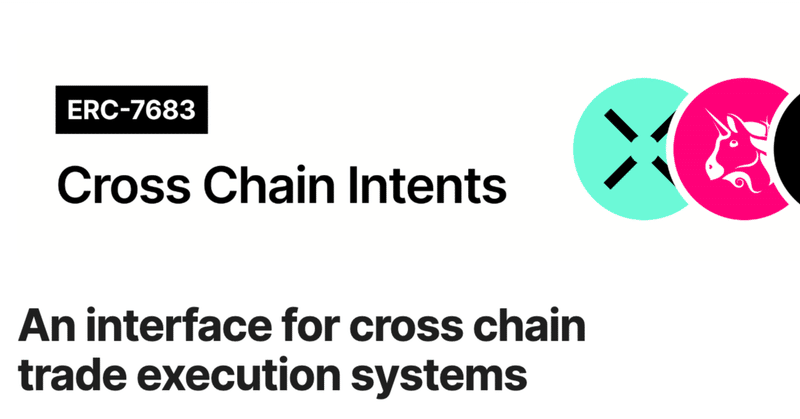
ERC-7683 クロスチェーンインテント|クロスチェーン取引実行システムのためのインターフェース
この記事は「An interface for cross chain trade execution systems」を日本語訳したものです。
Uniswap LabsとAcross は、クロスチェーン インテントの新しい標準を提案し、クロスチェーン アクションを指定するためのインテント ベース システムの統一フレームワークを確立します。
新しい標準の提案に伴い、Uniswap Labs と Across は共同でEthereum MagiciansフォーラムにEthereum Request for Commentを公開しました。2 つのプロジェクトは、 CAKE ワーキング グループに標準を提案し、議論とレビューを求めています。
Abstract(抽象化)
次の標準により、クロスチェーン取引実行システム用の標準 API の実装が可能になります。この標準では、汎用CrossChainOrder structと標準ISettlementContractスマート コントラクト インターフェイスが提供されます。
モチベーション
インテントベースのシステムは、従来のブリッジの複雑さと時間的制約を抽象化することで、エンドユーザーのクロスチェーンインタラクションの卓越したソリューションになりました。クロスチェーンインテントシステムの主な難しさの 1 つは、十分な流動性とチェーン全体のアクティブなフィラーのネットワークにアクセスすることです。この課題は、時間の経過とともに個別のチェーンの数が増えるにつれて悪化する可能性があります。その結果、コストの増加、待ち時間の増加、必要以上の失敗率の増加など、ユーザーエクスペリエンスが低下します。
標準を実装することで、クロスチェーン インテント システムは、order配信サービスやフィラー ネットワークなどのインフラストラクチャを相互運用して共有できるようになり、ユーザーのインテントを満たすための競争が激化してエンド ユーザー エクスペリエンスが向上します。
仕様
このドキュメント内のキーワード「MUST」、「MUST NOT」、「REQUIRED」、「SHALL」、「SHALL NOT」、「SHOULD」、「SHOULD NOT」、「RECOMMENDED」、「MAY」、「OPTIONAL」は、RFC 2119 で説明されているとおりに解釈されます。
CrossChainOrder struct
準拠したクロスチェーンorderタイプは、CrossChainOrderタイプに ABI デコード可能である必要があります。
/// @title CrossChainOrder type
/// @notice Standard order struct to be signed by swappers, disseminated to fillers, and submitted to settlement contracts
struct CrossChainOrder {
/// @dev The contract address that the order is meant to be settled by.
/// Fillers send this order to this contract address on the origin chain
address settlementContract;
/// @dev The address of the user who is initiating the swap,
/// whose input tokens will be taken and escrowed
address swapper;
/// @dev Nonce to be used as replay protection for the order
uint256 nonce;
/// @dev The chainId of the origin chain
uint32 originChainId;
/// @dev The timestamp by which the order must be initiated
uint32 initiateDeadline;
/// @dev The timestamp by which the order must be filled on the destination chain
uint32 fillDeadline;
/// @dev Arbitrary implementation-specific data
/// Can be used to define tokens, amounts, destination chains, fees, settlement parameters,
/// or any other order-type specific information
bytes orderData;
}
この標準を実装するクロスチェーン実行システムは、任意のフィールドから解析できるカスタム サブタイプを作成する必要がorderDataあります。これには、スワップに関係するトークン、宛先チェーン ID、フルフィルメント制約、決済オラクルなどの情報が含まれる場合があります。
ResolvedCrossChainOrder struct
準拠したクロスチェーンorderタイプは、ResolvedCrossChainOrderstructに変換可能である必要があります。
/// @title ResolvedCrossChainOrder type
/// @notice An implementation-generic representation of an order
/// @dev Defines all requirements for filling an order by unbundling the implementation-specific orderData.
/// @dev Intended to improve integration generalization by allowing fillers to compute the exact input and output information of any order
struct ResolvedCrossChainOrder {
/// @dev The contract address that the order is meant to be settled by.
address settlementContract;
/// @dev The address of the user who is initiating the swap
address swapper;
/// @dev Nonce to be used as replay protection for the order
uint256 nonce;
/// @dev The chainId of the origin chain
uint32 originChainId;
/// @dev The timestamp by which the order must be initiated
uint32 initiateDeadline;
/// @dev The timestamp by which the order must be filled on the destination chain(s)
uint32 fillDeadline;
/// @dev The inputs to be taken from the swapper as part of order initiation
Input[] swapperInputs;
/// @dev The outputs to be given to the swapper as part of order fulfillment
Output[] swapperOutputs;
/// @dev The outputs to be given to the filler as part of order settlement
Output[] fillerOutputs;
}
/// @notice Tokens sent by the swapper as inputs to the order
struct Input {
/// @dev The address of the ERC20 token on the origin chain
address token;
/// @dev The amount of the token to be sent
uint256 amount;
}
/// @notice Tokens that must be receive for a valid order fulfillment
struct Output {
/// @dev The address of the ERC20 token on the destination chain
/// @dev address(0) used as a sentinel for the native token
address token;
/// @dev The amount of the token to be sent
uint256 amount;
/// @dev The address to receive the output tokens
address recipient;
/// @dev The destination chain for this output
uint32 chainId;
}
ISettlementContract インターフェース
準拠した決済契約の実装では、次のISettlementContractインターフェースを実装する必要があります。
/// @title ISettlementContract
/// @notice Standard interface for settlement contracts
interface ISettlementContract {
/// @notice Initiates the settlement of a cross-chain order
/// @dev To be called by the filler
/// @param order The CrossChainOrder definition
/// @param signature The swapper's signature over the order
/// @param fillerData Any filler-defined data required by the settler
function initiate(CrossChainOrder order, bytes signature, bytes fillerData) external;
/// @notice Resolves a specific CrossChainOrder into a generic ResolvedCrossChainOrder
/// @dev Intended to improve standardized integration of various order types and settlement contracts
/// @param order The CrossChainOrder definition
/// @param fillerData Any filler-defined data required by the settler
/// @returns ResolvedCrossChainOrder hydrated order data including the inputs and outputs of the order
function resolve(CrossChainOrder order, bytes fillerData) external view returns (ResolvedCrossChainOrder);
}
根拠
Generic OrderData
重要な考慮事項は、幅広いクロスチェーン インテント設計が同じ標準内で機能できるようにすることです。これを実現するために、仕様は標準的なクロスチェーン インテントフローを中心として設計され、そのフロー内でさまざまな実装の詳細を許可しています。
標準的なクロスチェーン インテント フロー:
スワッパーはorderのパラメータを定義するオフチェーンメッセージに署名する
orderは充填業者に配布される
フィラーは原点チェーン上で取引を開始する
フィラーは宛先チェーンのorderを満たす
orderを決済するためにクロスチェーン決済プロセスが行われる
このフローでは、標準の実装者は次のような動作をカスタマイズするための設計柔軟性が得られます。
価格決定、例えばダッチオークションやオラクルベースの価格設定
履行制約
決済手続き
このorderDataフィールドにより、実装ではこれらの動作に対して任意の仕様を採用できるようになり、同時にインテグレーターはorderのプライマリ フィールドを解析できるようになります。
この機能は、resolveビュー機能とタイプにも影響を与えました。解決により、フィラーを統合して、手元のorderDataフィールドResolvedCrossChainOrderに関する特定の知識がなくてもorderを検証および評価できるようになります。
この機能は、resolveビュー関数と ResolvedCrossChainOrder タイプにも影響を与えました。 Resolution により、integrating fillersは、orderData フィールドに関する具体的な知識がなくても、orderを検証および評価できるようになります。
Permit2の使用
Permit2 はこの標準では特に必須ではありませんが、標準に準拠したプロトコルを構築するための効率的で簡単なアプローチを提供します。具体的には、witnesspermit2 の機能により、スワッパーは単一の署名でトークン転送とorder自体の両方を承認できます。これにより、トークンの転送とorderの正常な開始がうまく結び付けられます。
対照的に、標準的な承認モデルでは、トークンの承認とスワップの条件を承認するための署名という 2 つの別々の署名が必要になります。また、トークンの承認とスワップが切り離されるため、承認されたトークンは、バグのある、または信頼できないsettlerコントラクトのためにいつでも取得される可能性があります。
Permit2の周囲に標準準拠のセトラーシステムを構築する場合、以下の点を考慮する必要があります。
nonce order structはpermit2 nonceである必要があります
initiateDeadline order structにはpermit2の期限が必要です
解析された完全なorder structは、orderDatapermit2 呼び出し中にwitnessタイプとして使用する必要があります。これにより、スワッパーがorder許可に署名する際に最大限の透明性が確保されます。
この記事が気に入ったらサポートをしてみませんか?