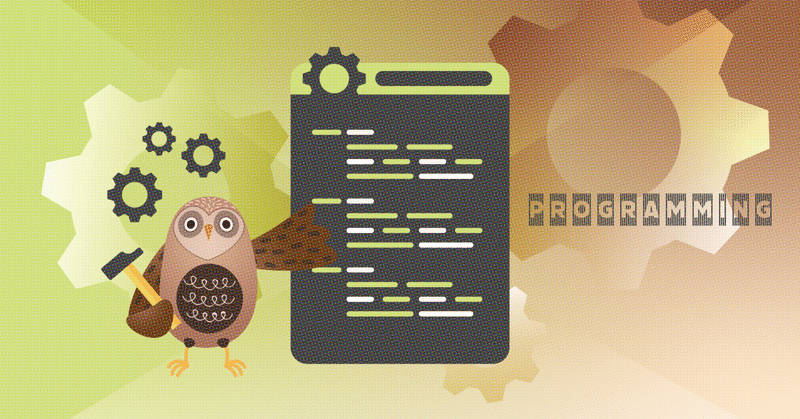
Photo by
ia19200102
HTML5レベル2 勉強メモ: グラフィックス・アニメーション
ビットマップ形式とベクター形式の描画
ビットマップ形式:画像をピクセル単位で表現する形式
・各ピクセルは特定の色を持つ
・拡大すると画像が粗くなる
・代表的な例は、JPEG, GIF< ProcessingInstruction, BMP
・フォトショなどで使われる
ベクター形式:画像を図形の集合で表現する形式
・画像を線、曲線、円などで表現する
・拡大しても画像が粗くならない
・代表的な例はSVG, EPS, PDFなど
・イラストレーターなどで使われる
Canvasの特徴
・ビットマップ形式(ピクセルの集合)で描画する
・JavaScriptからCanvas上のピクセルを描画できる
・描画した図形を画像ファイルに書き出せる
・アニメーション描画用のメソッドはない
CanvasRenderingContext2D オブジェクト
Canvas要素を操作するためのAPI
HTMLのcanvas要素を取得し、getContext('2d')メソッドで描画のためのオブジェクトを取得できる
図家の描画を行うプロパティやメソッドがある
globalAlpha
globalCompositeOperation
font
fillStyle
strokeStyle
beginPath()
closePath()
sava()
restore()
rect()
clip()
moveTo()
lineTo()
stroke()
fillRect()
strokeRect()
clearRect()
arc()
arcTo()
bezierCurveTo()
quadraticCurveTo()
measureText()
fillText()
strokeText()
setTransform()
rotate()
scale()
translate()
drawImage()
createImageData()
// Canvas要素を取得し、2Dレンダリングコンテキストを作成します
var canvas = document.getElementById('myCanvas');
var ctx = canvas.getContext('2d');
// プロパティの使用例
ctx.globalAlpha = 0.5; // 透明度を設定します
ctx.globalCompositeOperation = 'multiply'; // 合成操作を設定します
ctx.font = '30px Arial'; // フォントを設定します
ctx.fillStyle = 'red'; // 塗りつぶし色を設定します
ctx.strokeStyle = 'blue'; // 線の色を設定します
// メソッドの使用例
ctx.beginPath(); // 新しいパスを開始します
ctx.moveTo(50, 50); // パスを指定した座標に移動します
ctx.lineTo(100, 100); // 現在の座標から指定した座標まで線を描きます
ctx.closePath(); // パスを閉じます
ctx.stroke(); // 現在のパスを描きます
ctx.fillRect(50, 50, 100, 100); // 指定した座標に塗りつぶしの四角形を描きます
ctx.strokeRect(50, 50, 100, 100); // 指定した座標に線の四角形を描きます
ctx.clearRect(50, 50, 100, 100); // 指定した座標の四角形をクリアします
ctx.arc(50, 50, 50, 0, Math.PI * 2, false); // 円を描きます
ctx.arcTo(50, 50, 100, 100, 50); // 円弧を描きます
ctx.bezierCurveTo(50, 50, 100, 100, 150, 150); // ベジェ曲線を描きます
ctx.quadraticCurveTo(50, 50, 100, 100); // 二次曲線を描きます
var textMetrics = ctx.measureText('Hello World'); // テキストのメトリクスを取得します
ctx.fillText('Hello World', 50, 50); // テキストを塗りつぶしで描きます
ctx.strokeText('Hello World', 50, 50); // テキストを線で描きます
ctx.setTransform(1, 0, 0, 1, 0, 0); // 変換行列を設定します
ctx.rotate(Math.PI / 180 * 45); // 回転します
ctx.scale(2, 2); // スケーリングします
ctx.translate(50, 50); // 移動します
var img = new Image();
img.onload = function() {
ctx.drawImage(img, 0, 0); // 画像を描きます
};
img.src = 'image.jpg';
var imageData = ctx.createImageData(100, 100); // 新しいImageDataオブジェクトを作成します
基本的な図形の描画例
// Canvas要素を取得し、2Dレンダリングコンテキストを作成します
var canvas = document.getElementById('myCanvas');
var ctx = canvas.getContext('2d');
// 四角形を描画します
ctx.fillStyle = 'red';
ctx.fillRect(50, 50, 100, 100);
// 丸を描画します
ctx.beginPath();
ctx.arc(250, 100, 50, 0, Math.PI * 2, false);
ctx.fillStyle = 'blue';
ctx.fill();
// 三角形を描画します
ctx.beginPath();
ctx.moveTo(400, 150);
ctx.lineTo(450, 50);
ctx.lineTo(500, 150);
ctx.closePath();
ctx.fillStyle = 'green';
ctx.fill();
// 四角形を回転させます
ctx.save();
ctx.translate(100, 100);
ctx.rotate(Math.PI / 180 * 45);
ctx.fillRect(-50, -50, 100, 100);
ctx.restore();
// 丸を移動します
ctx.beginPath();
ctx.arc(250 + 100, 100, 50, 0, Math.PI * 2, false);
ctx.fillStyle = 'blue';
ctx.fill();
// 三角形を回転させて移動します
ctx.save();
ctx.translate(450, 100);
ctx.rotate(Math.PI / 180 * 30);
ctx.beginPath();
ctx.moveTo(-50, 50);
ctx.lineTo(0, -50);
ctx.lineTo(50, 50);
ctx.closePath();
ctx.fillStyle = 'green';
ctx.fill();
ctx.restore();
canvas要素
WebGL API, Canvas APIでアニメーションやグラフィックスを表示する。
・Canvas要素だけでは何も表示されない。
・<script>で設定する必要がある。
・width, heightを指定できる
・src属性はない
<canvas id="myCanvas" width="300" height="150" style="border:1px solid #000 ;"></canvas>
<script>
// Get the canvas element
var canvas = document.getElementById("myCanvas");
// Get the drawing context
var ctx = canvas.getContext("2d");
// Draw a red rectangle
ctx.fillStyle = "red";
ctx.fillRect(20, 20, 100, 50);
// Draw a blue circle
ctx.beginPath();
ctx.arc(200, 75, 50, 0, 2 * Math.PI);
ctx.fillStyle = "blue";
ctx.fill();
ctx.closePath();
</script>
図形の書き方
この記事が気に入ったらサポートをしてみませんか?