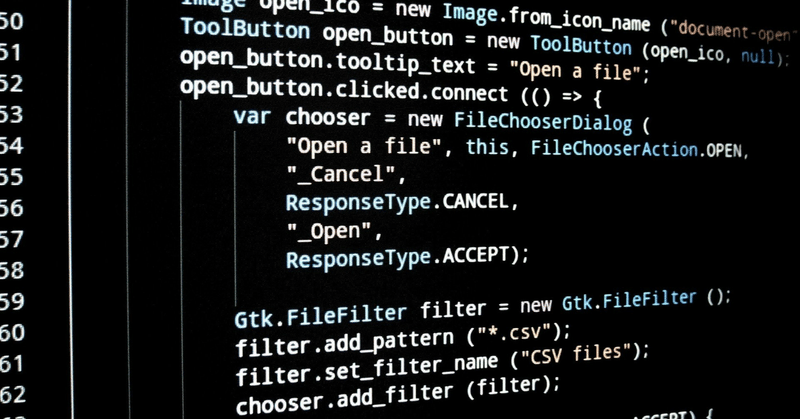
HTML5レベル2 勉強メモ: JavaScript API
イベント
Eventインタフェース
プロパティ:
Event.target:イベントが発生した要素を取得
Event.type:イベントの種類(例:click, keydown など)を取得
Event.currentTarget:現在処理中のイベントハンドラがアタッチされている要素を取得
Event.bubbles:イベントがバブリングフェーズであるかどうかを示すブール値を取得
メソッド:
Event.preventDefault():イベントのデフォルトのアクションをキャンセルする
Event.stopPropagation():イベントの伝播を停止
Event.stopImmediatePropagation():他のすべてのイベントリスナーによるイベントの処理を停止
document.getElementById('myButton').addEventListener('click', function(event) {
console.log(event.target); // クリックされた要素を出力
console.log(event.type); // "click" を出力
console.log(event.currentTarget); // イベントリスナーがアタッチされている要素を出力
console.log(event.bubbles); // true を出力(clickイベントはバブリングフェーズを持つ)
});
document.getElementById('myLink').addEventListener('click', function(event) {
event.preventDefault(); // リンクのデフォルトのアクション(ページ遷移)をキャンセル
// 何かの処理...
event.stopPropagation(); // イベントの伝播を停止(親要素へのイベントのバブリングを防ぐ)
// 何かの処理...
event.stopImmediatePropagation(); // 他のすべてのイベントリスナーによるイベントの処理を停止
});
イベントハンドラ
イベント発生時の処理を定義したもの
イベントハンドラの記述方法
HTMLのタグ内に記述
<input type="button" value="OK" onclick="alert('クリック');">
JavaScriptのコード内に記述する
window.onload = function() {
document.getElementById('btn').onclick = function() {
alert('クリック');
};
};
イベントリスナ
イベントハンドラと同様にイベント発生時の処理を定義できる
イベントハンドラとイベントリスナの違い
イベントハンドラは1つの要素に対して1つの処理しか設定できない
イベントリスナは複数の処理を設定できる
イベントハンドラは、新たにイベントハンドラを設定すると、既存のものは上書きされる
<button onclick="console.log('First handler')">Click me</button>
<script>
// この行は上記のonclickハンドラを上書きします
document.querySelector('button').onclick = function() { console.log('Second handler') };
</script>
let button = document.querySelector('button');
button.addEventListener('click', function() {
console.log('First listener');
});
button.addEventListener('click', function() {
console.log('Second listener');
});
<button>Click me</button>
windowオブジェクト
・ブラウザ上でのグローバルオブジェクト
・windowsオブジェクトのプロパティにアクセスする際、
「window.」を省略できる
・ブラウザのウィンドウを操作できる
・windowオブジェクト経由でDocumentオブジェクトにアクセスできる
プロパティ
console
document
indexedDB
location
localStorage/sessionStorage
navigator
worker
consoleの使用例:
console.log('Hello, world!'); // メッセージをコンソールに出力
documentの使用例:
let title = document.title; // ドキュメントのタイトルを取得
indexedDBの使用例:
let dbRequest = window.indexedDB.open('myDatabase', 1); // 新しいデータベースを開くまたは既存のデータベースに接続
locationの使用例:
let url = window.location.href; // 現在のURLを取得
localStorage/sessionStorageの使用例:
window.localStorage.setItem('key', 'value'); // localStorageにデータを保存
let value = window.localStorage.getItem('key'); // localStorageからデータを取得
window.sessionStorage.setItem('key', 'value'); // sessionStorageにデータを保存
value = window.sessionStorage.getItem('key'); // sessionStorageからデータを取得
navigatorの使用例:
let userAgent = window.navigator.userAgent; // ユーザーエージェントの文字列を取得
workerの使用例:
let myWorker = new Worker('worker.js'); // 新しいWeb Workerを作成
windowオブジェクトのメソッド
alert(): ユーザーにアラートメッセージを表示
window.alert('Hello, world!');
setTimeout(): 指定した時間が経過した後に関数を実行
window.setTimeout(function() {
console.log('Hello, world!');
}, 2000); // 2秒後にメッセージを出力
clearTimeout(): setTimeout() によって設定されたタイマーをキャンセル
let timeoutId = window.setTimeout(function() {
console.log('Hello, world!');
}, 2000);
window.clearTimeout(timeoutId); // タイマーをキャンセル
setInterval(): 指定した時間間隔ごとに関数を実行
window.setInterval(function() {
console.log('Hello, world!');
}, 2000); // 2秒ごとにメッセージを出力
clearInterval(): setInterval() によって設定されたタイマーをキャンセル
let intervalId = window.setInterval(function() {
console.log('Hello, world!');
}, 2000);
window.clearInterval(intervalId); // タイマーをキャンセル
fetch(): リソースをネットワークから取得
window.fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
confirm: ユーザーに確認メッセージを表示し、OKまたはキャンセルのボタンをクリックするまでスクリプトの実行を停止
ユーザーがOKをクリックすると true を、キャンセルをクリックすると false を返す
let result = window.confirm('Are you sure you want to continue?');
if (result) {
console.log('User clicked OK');
} else {
console.log('User clicked Cancel');
}
prompt: ユーザーにテキスト入力を求めるダイアログを表示
ユーザーがOKをクリックすると入力したテキストを、キャンセルをクリックすると null を返す。
let name = window.prompt('What is your name?');
if (name) {
console.log(`Hello, ${name}!`);
} else {
console.log('User did not enter a name');
}
open: 新しいブラウザウィンドウを開く
URL、ウィンドウ名、およびウィンドウの特性を指定する
window.open('https://www.example.com', '_blank', 'width=500,height=500');
window.postMessage: 異なるオリジン間で安全にデータを送信する
まず新しいウィンドウを開き、その後 postMessage メソッドを使用してそのウィンドウにメッセージを送信。
第一引数は送信するメッセージ、第二引数はメッセージを受信するウィンドウのオリジン(スキーム、ホスト、ポート)
// 新しいウィンドウを開く
let newWindow = window.open('https://www.example.com');
// 新しいウィンドウにメッセージを送信
newWindow.postMessage('Hello, world!', 'https://www.example.com');
受信側のウィンドウでは、message イベントリスナーを設定してメッセージを受信
window.addEventListener('message', function(event) {
// オリジンをチェックして、期待するオリジンからのメッセージのみを受信する
if (event.origin !== 'http://example.org') return;
console.log('Received message:', event.data);
});
window.setInterval(): 指定した間隔(ミリ秒)ごとに関数を繰り返し実行
let counter = 0;
let intervalId = window.setInterval(function() {
counter++;
console.log(`Interval has run ${counter} times`);
if (counter === 5) {
window.clearInterval(intervalId); // 5回実行したらインターバルをクリア
}
}, 1000); // 1秒ごとに関数を実行
window.setTimeout(): 指定した時間(ミリ秒)が経過した後に一度だけ関数を実行
window.setTimeout(function() {
console.log('Timeout has run');
}, 2000); // 2秒後に関数を実行
ブラウザのサイズを変更した際のWindowオブジェクト
change
devicemotion
deviceorientation
hashchange
load
message
popstate
resize
scroll
storage
resizeの使用例:
ブラウザウィンドウのサイズが変更されたときに発生
window.addEventListener('resize', function(event) {
console.log('Window was resized');
});
loadの使用例:
ページ全体(画像やスクリプトを含む)が完全に読み込まれたときに発生
window.addEventListener('load', function(event) {
console.log('Page fully loaded');
});
scrollの使用例:
ユーザーがページをスクロールすると発生
window.addEventListener('scroll', function(event) {
console.log('User scrolled the page');
});
hashchangeの使用例:
URLのハッシュ(# 以降の部分)が変更されたときに発生
window.addEventListener('hashchange', function(event) {
console.log('URL hash changed');
});
storageの使用例:
ローカルストレージが変更されたときに発生
window.addEventListener('storage', function(event) {
console.log('Local storage changed');
});
messageの使用例:
他のウィンドウからメッセージが送信されたときに発生
window.addEventListener('message', function(event) {
console.log('Received message:', event.data);
});
popstateの使用例:
履歴エントリが変更されたとき(ユーザーがブラウザの戻るまたは進むボタンをクリックしたとき)に発生
window.addEventListener('popstate', function(event) {
console.log('History entry changed');
});
ブラウザの座標情報
innerHeight
innerWidth
outerHeight
outerWidth
pageXOffset
pageYOffset
screeX
screenY
scrollTo
// innerHeight: ブラウザウィンドウのビューポートの高さ(ピクセル)
console.log('innerHeight:', window.innerHeight);
// innerWidth: ブラウザウィンドウのビューポートの幅(ピクセル)
console.log('innerWidth:', window.innerWidth);
// outerHeight: ブラウザウィンドウ全体の高さ(ピクセル)
console.log('outerHeight:', window.outerHeight);
// outerWidth: ブラウザウィンドウ全体の幅(ピクセル)
console.log('outerWidth:', window.outerWidth);
// pageXOffset: ページが水平方向にスクロールしたピクセル数
console.log('pageXOffset:', window.pageXOffset);
// pageYOffset: ページが垂直方向にスクロールしたピクセル数
console.log('pageYOffset:', window.pageYOffset);
// screenX: ブラウザウィンドウの左上隅がスクリーンの左上隅からどれだけ離れているか(ピクセル)
console.log('screenX:', window.screenX);
// screenY: ブラウザウィンドウの左上隅がスクリーンの上端からどれだけ離れているか(ピクセル)
console.log('screenY:', window.screenY);
// ページの最上部にスクロール
window.scrollTo(0, 0);
// ページの特定の位置(例えば、x: 100px, y: 200px)にスクロール
window.scrollTo(100, 200);
// スムーズにページの最上部にスクロール
window.scrollTo({ top: 0, behavior: 'smooth' });
// スムーズにページの特定の位置(例えば、y: 200px)にスクロール
window.scrollTo({ top: 200, behavior: 'smooth' });
windowオブジェクトのブラウザ操作のメソッド
close()
moveBy()
moveTo()
open(9
resizeBy()
resizeTo()
window.close()
現在のブラウザウィンドウを閉じる
window.close();
window.moveBy()
ブラウザウィンドウを指定したピクセルだけ移動する
// 現在の位置から右に100ピクセル、下に50ピクセル移動
window.moveBy(100, 50);
window.moveTo()
ブラウザウィンドウを指定した位置に移動する
// スクリーンの左上隅にウィンドウを移動
window.moveTo(0, 0);
window.open()
新しいブラウザウィンドウを開く
// 新しいウィンドウでGoogleのホームページを開く
window.open('https://www.google.com', '_blank');
window.resizeBy()
ブラウザウィンドウのサイズを指定したピクセルだけ変更
// 現在のサイズから幅を100ピクセル、高さを50ピクセル増やす
window.resizeBy(100, 50);
window.resizeTo()
ブラウザウィンドウのサイズを指定したピクセルに変更
// ウィンドウのサイズを幅500ピクセル、高さ300ピクセルに設定
window.resizeTo(500, 300);
document.querySelector() 引数で指定したセレクタに一致するHTML要素を返す
タイプセレクタ、クラスセレクタ、IDセレクタ、属性セレクタ、複合セレクタなど、さまざまなセレクタを指定できる
// 最初の <p> 要素を選択
let p = document.querySelector('p');
// 最初の .myClass クラスを持つ要素を選択
let myClass = document.querySelector('.myClass');
// idが 'myId' の要素を選択
let myId = document.querySelector('#myId');
// 最初の data-custom-attribute 属性を持つ要素を選択
let customAttribute = document.querySelector('[data-custom-attribute]');
// 最初の <div> 要素で、.myClass クラスを持ち、idが 'myId' の要素を選択
let compound = document.querySelector('div.myClass#myId');
document.querySelectorAll("input");
セレクタが存在しない場合、または間違ったセレクタが指定された場合、null が返る
let element = document.querySelector('#nonexistentId'); // 存在しないID
console.log(element); // null
ID名があっていても、その要素が value 属性を持たない場合(例えば、<div> や <p> などの要素)、element.value は undefined が返る
let element = document.querySelector('#myDiv'); // <div id="myDiv"></div>
console.log(element.value); // undefined
value 属性を持つ可能性がある要素(例えば、<input> や <select> など)で value が設定されていない場合、element.value は空文字列("")を返す
let element = document.querySelector('#myInput'); // <input id="myInput">
console.log(element.value); // ""
document.querySelectorAll() 引数で指定したセレクタに一致するすべてのHTML要素を返す
・document.querySelectorAll() を使う
・複数のinputとボタンがある
・いずれのinputにも値がない場合はクリック押下時にアラートが表示される
<input type="text" class="input-field">
<input type="text" class="input-field">
<button id="myButton">Click me</button>
document.getElementById('myButton').addEventListener('click', function() {
let inputs = document.querySelectorAll('input');
let isEmpty = Array.from(inputs).every(input => input.value === '');
if (isEmpty) {
alert('All input fields are empty!');
}
});
getElementById()とquerySelector()のセレクタの指定 注意
<div id="target">Hello</div>
#をつけない
document.getElementById('target'); // <div id="target">Hello</div>
#をつける
document.querySelector('#target'); // <div id="target">Hello</div>
Historyオブジェクト
ブラウザの履歴を操作するオブジェクト
履歴の追加、変更、履歴を通してページの移動ができる
画面遷移をしないシングルページアプリで状態を管理できる
Historyオブジェクトのプロパティ、メソッド
length
state
go()
back()
forward()
pushState()
replaceState()
history.length
ブラウザの履歴スタックの長さを返す
console.log(history.length); // ブラウザの履歴スタックの長さを表示
history.state
現在の履歴エントリの状態オブジェクトを返す
console.log(history.state); // 現在の履歴エントリの状態オブジェクトを表示
history.go()
ブラウザの履歴スタック内を移動
history.go(-1); // 一つ前の履歴エントリに移動
history.go(1); // 一つ次の履歴エントリに移動
history.back()
一つ前の履歴エントリに移動
history.back(); // 一つ前の履歴エントリに移動
history.forward()
一つ次の履歴エントリに移動
history.forward(); // 一つ次の履歴エントリに移動
history.pushState()
履歴スタックに新しいエントリを追加
第1引数は履歴に追加する任意のデータ
第2引数は履歴のタイトル、これはなんでもいい。ほとんどのブラウザでは無視されるので(省略不可なので必要)
第2引数は履歴のURL
history.pushState({page: 1}, "title", "?page=1"); // 履歴スタックに新しいエントリを追加
元のURL: http://www.example.com
// 現在のURLに対して新しい履歴エントリを追加
let stateObj = { foo: "bar" };
history.pushState(stateObj, "page 2", "page2.html");
pushState()を使った後、http://www.example.com/page2.html
相対パスを使えばオリジンは同じになる
元のURLは http://localhost/index
// 今のURLの末尾が変わる
window.onload = function () {
history.pushState({data: "foo"}, "title", "foo"); // http://localhost/foo
}
// 末尾に足していく
window.onload = function () {
history.pushState({data: "foo"}, "title", "index/foo"); // http://localhost/index/foo
}
window.onload = function () {
history.pushState({data: "foo"}, "foo", "foo"); // http://localhost/foo
history.pushState({data: "bar"}, "bar", "bar"); // http://localhost/bar
history.pushState({data: "foge"}, "foge", "foge"); // http://localhost/foge
}
window.onload = function () {
history.pushState({data: "foo"}, "foo", "index/foo"); // http://localhost/index/foo
history.pushState({data: "bar"}, "bar", "index/foo/bar"); // http://localhost/index/foo/bar
history.pushState({data: "foge"}, "foge", "index/foo/bar/foge"); // http://localhost/index/foo/bar/foge
history.pushState({data: "foge"}, "foge", "http://localhost/unindexed/aaaaa/bbbbb"); // http://localhost/unindexed/aaaaa/bbbbb
history.pushState({data: "foge"}, "foge", "http://localhost:8000/index.html"); // オリジンが異なるのでエラーが発生
}
history.replaceState()
現在の履歴エントリを新しいエントリで置き換え
history.replaceState({page: 2}, "title", "?page=2"); // 現在の履歴エントリを新しいエントリで置き換え
http://www.example.com/page2.html を replaceState() を使用して新しいURLで置き換え
// 現在のURLを新しいURLで置き換え
let stateObj = { foo: "baz" };
history.replaceState(stateObj, "page 3", "page3.html");
最終的なURLは http://www.example.com/page3.html
pushState()とgo()を使って履歴を操作
履歴は現在の履歴エントリを基準に数える
このメソッドに正の数を渡すと、その数だけ前方の履歴エントリに移動
例えば、history.go(1) は history.forward() と同等で、一つ次の履歴エントリに移動
逆に、このメソッドに負の数を渡すと、その数だけ後方の履歴エントリに移動
例えば、history.go(-1) は history.back() と同等で、一つ前の履歴エントリに移動
history.go(0) または history.go() を呼び出すと、現在のページがリロードされる
// 現在のURLに対して新しい履歴エントリを追加
// 現在のURL(例えば、http://www.example.com)
let stateObj = { foo: "bar" };
history.pushState(stateObj, "page 2", "page2.html"); // http://www.example.com/page2.html
// 一つ前の履歴エントリに移動
history.go(-1); // http://www.example.com
let stateObj = { foo: "bar" };
history.pushState(stateObj, "page 2", "page2.html"); // http://www.example.com/page2.html
history.go(1); // 前方に移動する履歴エントリがないため、何も起こらない
popState
window.onload = function () {
history.pushState({data: "foo"}, "foo", "foo"); // http://localhost/foo
history.pushState({data: "bar"}, "bar", "bar"); // http://localhost/bar
history.back(); // http://localhost/foo
history.state; // {data: "foo"}
}
Location オブジェクト
URLを操作するオブジェクト
Locationオブジェクトのプロパティ、メソッド
href
protocol
host
hostname
port
pathname
search
hash
assign()
replace()
reload()
// href: 現在のページの完全なURLを取得または設定します
console.log(location.href); // "http://www.example.com:8080/pathname?search=test#hash"
location.href = "http://www.google.com";
// protocol: 現在のURLのプロトコル部分を取得または設定します
console.log(location.protocol); // "http:"
location.protocol = "https";
// host: 現在のURLのホスト名とポート番号を取得または設定します
console.log(location.host); // "www.example.com:8080"
location.host = "www.google.com:8080";
// hostname: 現在のURLのホスト名を取得または設定します
console.log(location.hostname); // "www.example.com"
location.hostname = "www.google.com";
// port: 現在のURLのポート番号を取得または設定します
console.log(location.port); // "8080"
location.port = "8080";
// pathname: 現在のURLのパス部分を取得または設定します
console.log(location.pathname); // "/pathname"
location.pathname = "/newPathname";
// search: 現在のURLのクエリ文字列部分を取得または設定します
console.log(location.search); // "?search=test"
location.search = "?newSearch=newValue";
// hash: 現在のURLのフラグメント識別子を取得または設定します
console.log(location.hash); // "#hash"
location.hash = "#newHash";
// assign(): 新しいURLをロードします
location.assign("http://www.google.com");
// replace(): 現在のURLを新しいURLで置き換え、現在のページを履歴から削除します
location.replace("http://www.google.com");
// reload(): 現在のページをリロードします
location.reload();
Consoleオブジェクト
ブラウザのコンソールに情報を出力する機能を提供するオブジェクト
assert()
clear()
debug()
dir()
dirxml()
error()
info()
log()
profile()
profileEnd()
trace()
warn()
// assert(): 指定した条件がfalseの場合にメッセージを表示します
console.assert(false, 'This is an error message'); // Output: Assertion failed: This is an error message
// clear(): コンソールの出力をクリアします
console.clear();
// debug(): デバッグメッセージを表示します
console.debug('Debug message'); // Output: Debug message
// dir(): 指定したJavaScriptオブジェクトのプロパティを表示します
console.dir(document.location); // Output: Displays the properties of the document.location object
// dirxml(): XML/HTML要素をテキストとして表示します
console.dirxml(document.body); // Output: Displays the HTML of the body element
// error(): エラーメッセージを表示します
console.error('Error message'); // Output: Error message
// info(): 情報メッセージを表示します
console.info('Info message'); // Output: Info message
// log(): ログメッセージを表示します
console.log('Log message'); // Output: Log message
// profile(): JavaScriptのCPUプロファイルを開始します
console.profile('My profile');
// profileEnd(): 最後に開始したCPUプロファイルを終了します
console.profileEnd('My profile');
// trace(): スタックトレースを表示します
console.trace('Show trace'); // Output: Show trace
// warn(): 警告メッセージを表示します
console.warn('Warning message'); // Output: Warning message
プロファイラ
各処理の実行時間などを記録し、パフォーマンスの改善に使うツール
MessageEvent オブジェクト
window.addEventListener('message', function(event) {
// dataプロパティは送信されたメッセージを含みます
console.log('Received message:', event.data);
// originプロパティはメッセージの送信元のオリジン(スキーム、ホスト、ポート)を含みます
console.log('Message origin:', event.origin);
// sourceプロパティはメッセージの送信元のウィンドウを参照します
if (event.source === window.opener) {
console.log('Message came from the window that opened this one');
}
});
load イベント
イベントとはページ上で発生する様々な動作のこと
例えば、ページが読み込まれたときに発生する load イベントは、ページが読み込まれたときに発生する。
このイベントは、ページが読み込まれたときに実行したい処理を記述するのに使われる
イベントドリブンモデルとは、イベントに応じた処理を実行するモデルの事
・Documentオブジェクトはonloadイベントハンドラをプロパティとして持つ
・Windowオブジェクトのloadイベント発火時には、ページ内の全ての画像は読み込まれている
・Windowオブジェクトのloadイベント発火時には、ページ内の全てのDOMは読み込まれている
・loadイベントはページがキャッシュから読み込まれた場合は発火しない
フォームに関するイベント
blur
change
contextmenu
focus
input
invalid
Selectorssubmit
formchange
fomrinput
blur:要素からフォーカスが外れたときに発生
document.getElementById('myInput').addEventListener('blur', function() {
console.log('Input field lost focus');
});
change:要素の値が変更され、その要素からフォーカスが外れたときに発生
document.getElementById('myInput').addEventListener('change', function() {
console.log('Input field value changed');
});
contextmenu:要素上で右クリック(コンテキストメニューの呼び出し)が行われたときに発生
document.getElementById('myInput').addEventListener('contextmenu', function() {
console.log('Context menu opened on input field');
});
focus:要素がフォーカスを得たときに発生
document.getElementById('myInput').addEventListener('focus', function() {
console.log('Input field gained focus');
});
input:要素の値がユーザーによって変更されたときに発生
document.getElementById('myInput').addEventListener('input', function() {
console.log('User typed in input field');
});
invalid:要素が無効な状態になったとき(例えば、フォームのバリデーションに失敗したとき)に発生
document.getElementById('myForm').addEventListener('invalid', function() {
console.log('Form validation failed');
}, true);
キーボード操作に関するイベント
keydown
keypress
keyup
keydown:キーボードのキーが押されたときに発生
window.addEventListener('keydown', function(event) {
console.log('Key down: ' + event.key);
});
keypress:キーボードのキーが押され、文字が入力されたときに発生
window.addEventListener('keypress', function(event) {
console.log('Key press: ' + event.key);
});
keyup:キーボードのキーが離されたときに発生
window.addEventListener('keyup', function(event) {
console.log('Key up: ' + event.key);
});
キーボードイベント
主なプロパティ
code
キーコードを取得する
key
キーの値を取得できる
window.addEventListener('keydoown', function(e) {
console.log(e.code + e.key);
}, false);
マウス操作に関するイベント
click
dbclick
drag
dragend
dragenter
dragleave
dragover
dragstart
drop
mousedown
mousemove
mouseout
mouseover
mouseup
mousewheel
scroll
click:要素がクリックされたときに発生
document.getElementById('myElement').addEventListener('click', function() {
console.log('Element clicked');
});
dblclick:要素がダブルクリックされたときに発生
document.getElementById('myElement').addEventListener('dblclick', function() {
console.log('Element double-clicked');
});
drag:要素がドラッグされている間、継続的に発生
document.getElementById('myElement').addEventListener('drag', function() {
console.log('Element is being dragged');
});
dragend:ユーザーが要素のドラッグを終了したときに発生
document.getElementById('myElement').addEventListener('dragend', function() {
console.log('Element drag ended');
});
dragenter:ドラッグされた要素がドロップターゲットに入ったときに発生
document.getElementById('myElement').addEventListener('dragenter', function() {
console.log('Dragged element entered drop target');
});
dragleave:ドラッグされた要素がドロップターゲットから出たときに発生
document.getElementById('myElement').addEventListener('dragleave', function() {
console.log('Dragged element left drop target');
});
dragover:ドラッグされた要素がドロップターゲットの上にある間、継続的に発生
document.getElementById('myElement').addEventListener('dragover', function() {
console.log('Dragged element is over drop target');
});
dragstart:ユーザーが要素のドラッグを開始したときに発生
document.getElementById('myElement').addEventListener('dragstart', function() {
console.log('Element drag started');
});
drop:ドラッグされた要素がドロップターゲットにドロップされたときに発生
document.getElementById('myElement').addEventListener('drop', function() {
console.log('Dragged element dropped on drop target');
});
mousedown:ユーザーがマウスボタンを押したときに発生
document.getElementById('myElement').addEventListener('mousedown', function() {
console.log('Mouse button pressed down');
});
mousemove:マウスポインタが要素の上を移動するときに発生
document.getElementById('myElement').addEventListener('mousemove', function() {
console.log('Mouse pointer moved over element');
});
mouseout:マウスポインタが要素から出たときに発生
document.getElementById('myElement').addEventListener('mouseout', function() {
console.log('Mouse pointer left element');
});
mouseover:マウスポインタが要素の上に入ったときに発生
document.getElementById('myElement').addEventListener('mouseover', function() {
console.log('Mouse pointer entered element');
});
mouseup:ユーザーがマウスボタンを離したときに発生
document.getElementById('myElement').addEventListener('mouseup', function() {
console.log('Mouse button released');
});
mousewheel:ユーザーがマウスホイールを回したときに発生
document.getElementById('myElement').addEventListener('mousewheel', function() {
console.log('Mouse wheel scrolled');
});
scroll:要素がスクロールされたときに発生
document.getElementById('myElement').addEventListener('scroll', function() {
console.log('Element scrolled');
});
タッチ操作に関するイベント
touchstart
touchmove
touchend
touchstart:ユーザーがタッチスクリーンに触れたときに発生
document.getElementById('myElement').addEventListener('touchstart', function() {
console.log('Touch started');
});
touchmove:ユーザーがタッチスクリーン上で指を動かしたときに発生
document.getElementById('myElement').addEventListener('touchmove', function() {
console.log('Touch moved');
});
touchend:ユーザーがタッチスクリーンから指を離したときに発生
document.getElementById('myElement').addEventListener('touchend', function() {
console.log('Touch ended');
});
ボタンクリック時にfoo関数を呼ぶイベントリスナ
button.addEventListener('click', foo, true);
入れ子構造の要素のクリックイベントの伝播
inner要素をクリックしたとき、inner要素のクリックイベントが発火した後、outer要素のクリックイベントが発火する。
つまり、INNER OUTER と表示される
子要素、親要素の順でイベントが発火する
<div id="outer">
<div id="inner">Hello</div>
</div>
var outer = document.getElementById('outer');
outer.onclick = function () {
console.log("OUTER");
}
var inner = document.getElementById('inner');
inner.onclick = function () {
console.log("INNER");
}
カスタムイベントの例
// カスタムイベントの作成
let myEvent = new CustomEvent('myEvent', {
detail: {
message: 'This is a custom event'
}
});
// イベントリスナの追加
document.addEventListener('myEvent', function(e) {
console.log(e.detail.message); // "This is a custom event" を出力
});
// カスタムイベントの発火
document.dispatchEvent(myEvent);
カスタムイベント
・ボタンをクリックすると現在の日時がブラウザに表示される
・実行してから10秒ごとに現在の日時がブラウザに表示される
<input type="button" value="現在日時取得" id="btn">
<span id="time"></span>
var button = document.getElementById('btn');
button.addEventListener('click', displalyTime);
var event = new Event("click");
window.setInterval(function () {
button.dispatchEvent(event);
}, 10000);
function displalyTime() {
var now = new Date();
document.getElementById('time').textContent = now.toLocaleTimeString();
}
・テキストボックスからフォーカスが外れるとイベントが発生する
・テキストボックスが未入力の場合、アラートが表示される
<form method="post" action="/hoge">
<label for="foo">Text: </label>
<input type="text" id="foo">
<button>OK</button>
</form>
document.getElementById("foo").addEventListener("blur", function (e) {
if (e.target.value === "") {
alert("no input");
}
}, false);
フォーム送信のメソッド
submit()
フォームの送信を行う
reset()
フォームの全ての値をリセットする
<form id="myForm" action="http://example.com" method="post">
<button type="button" onclick="submitForm()">Submit</button>
</form>
function submitForm() {
let form = document.getElementById('myForm');
// フォームを送信
form.submit();
// フォームをリセット
form.reset();
}
DOM(Document Object Model)
W3Cが仕様を策定しているHTML,XML,CSSのためのプログラミングインタフェース
JavaScriptにより、HTML要素の生成、操作、XML属性の操作が可能
DOMはwindowオブジェクトのdocumentプロパティ経由で使用する
-> documentプロパティはグローバルオブジェクトではない
-> window.documentのように、
windowグローバルオブジェクト経由でアクセスする
ページローディングの順番
DOMContent -> image -> page の順にログが出力される
DOMContentイベントは、DOMツリーの構築が完了すると発生する
画像などリソースの読み込みは待たなくてもいいので、一番最初に表示される画像が読み込まれて、imageが表示される
windowオブジェクトのloadイベントは、ページ全体の読み込みが完了すると発生する
<img id="img" src="test.png">
<script>
var img = document.getElementById('img');
img.onlaod = function() {
console.log('image');
}
document.addEventListener("DOMContentLoaded", function() {
console.log('DOMContent');
});
window.onload = function () {
console.log("page");
}
</script>
document.formsプロパティ
全てのform要素を返すプロパティ
1) document.forms[インデックス]
2) document.forms.フォーム名
3) document.forms["form要素のname属性"]
<form action="foo" method="post" name="inputForm">
<input type="text" name="inputText">
</form>
document.forms[0]
document.forms.inputForm
document.forms["inputForm"]
document.getElementById() と document.formsプロパティ
document.getElementById("ID名")
document.forms.フォーム名
<form action="foo" method="POST" name="inputForm">
<input type="text" name="bar" id="foo">
</form>
document.getElementById('foo') // <input type="text" name="bar" id="foo">
document.forms.inputForm // <form action="foo" method="POST" name="inputForm">
Element要素
HTML要素の操作
Element要素のプロパティ、メソッド
children
innerHTML
parentElement
style
textContent
value
appendChild()
hasAttribute()
insertBefore()
remove()
removeAttribute()
setAttribute()
setAtttributeNode()
let element = document.getElementById('myElement');
console.log(element.children); // 子要素のHTMLCollectionを出力
console.log(element.innerHTML); // 要素の内部HTMLを出力
console.log(element.parentElement); // 親要素を出力
console.log(element.style); // 要素のスタイル情報を出力
console.log(element.textContent); // 要素のテキスト内容を出力
console.log(element.value); // input要素の場合、その値を出力
let parent = document.getElementById('parent');
let child = document.getElementById('child');
let newElement = document.createElement('div');
parent.appendChild(newElement); // 新しい子要素を追加
console.log(child.hasAttribute('class')); // class属性が存在するかどうかを出力
parent.insertBefore(newElement, child); // childの前にnewElementを挿入
child.remove(); // child要素を削除
child.removeAttribute('class'); // class属性を削除
child.setAttribute('class', 'myClass'); // class属性を設定
let attr = document.createAttribute('id');
attr.value = 'myId';
child.setAttributeNode(attr); // id属性を設定
例) Googleの後ろにa要素を追加
URLはhttp://www.hogehoge.com
文字列はHogeHoge
<div id="container">
<a href="http://www.google.com">Google</a>
</div>
var aElement = document.createElement("a");
aElement.setAttribute("href", "http://www.hogehoge.com");
aElement.textContent = "HogeHoge";
document.getElementById("container").appendChild(aElement);
<div id="container">
<span id="target">FLM</span>
</div>
var spanElement = document.createElement("span");
spanElement.textContent = "SE";
var divElekent = document.getElementById("container");
var targetElement = document.getElementById("target");
divElement.insertBefore(spanELement, targetElement);
appendChild(childNode): 指定した子ノードを要素の最後の子として追加
let parent = document.getElementById('parent');
let newElement = document.createElement('div');
parent.appendChild(newElement);
removeChild(childNode): 指定した子ノードを要素から削除
let parent = document.getElementById('parent');
let child = document.getElementById('child');
parent.removeChild(child);
replaceChild(newNode, oldNode): 要素内の既存の子ノードを新しいノードに置き換え
let parent = document.getElementById('parent');
let oldChild = document.getElementById('oldChild');
let newChild = document.createElement('div');
parent.replaceChild(newChild, oldChild);
cloneNode(deep): 要素の複製を作成します。deep パラメータが true の場合、子ノードも複製
let element = document.getElementById('element');
let clone = element.cloneNode(true);
value と textContent
value プロパティは、input、select、textarea などのフォーム要素の現在の値を取得または設定する
<input id="myInput" type="text" value="Initial Value">
<div id="myDiv">Initial Text Content</div>
// input要素のvalueプロパティの使用例
let inputElement = document.getElementById('myInput');
// 値を取得
let inputValue = inputElement.value;
console.log(inputValue); // "Initial Value" を出力
// 値を設定
inputElement.value = 'New Value';
console.log(inputElement.value); // "New Value" を出力
textContent プロパティは、要素のテキスト内容を取得または設定する
子要素のマークアップを無視し、すべての子要素のテキストを連結したものを返す
<input id="myInput" type="text" value="Initial Value">
<div id="myDiv">Initial Text Content</div>
// div要素のtextContentプロパティの使用例
let divElement = document.getElementById('myDiv');
// テキスト内容を取得
let textContent = divElement.textContent;
console.log(textContent); // "Initial Text Content" を出力
// テキスト内容を設定
divElement.textContent = 'New Text Content';
console.log(divElement.textContent); // "New Text Content" を出力
submitイベント時に、inputの値のnullチェックはtextContentではなくvalueを使う
input 要素の値をチェックする場合、value プロパティを使用する
textContent プロパティは input 要素には適用されない
document.getElementById('myForm').addEventListener('submit', function(event) {
let inputElement = document.getElementById('myInput');
// input要素の値がnullまたは空文字列であるかチェック
if (!inputElement.value) {
event.preventDefault(); // フォームの送信をキャンセル
alert('Input cannot be null or empty');
}
});
HTML要素を非表示にする
<form action="foo" method="POST" id="inputForm">
<input type="text" name="bar" id="foo">
</form>
単純に非表示にする
1)
document.getElementById("inputForm").setAttribute("class", "hidden");
2)
document.getElementById("inputForm").style = "display: none";
要素自体を削除する
3)
document.getElementById("inputForm").parentElement.innerHTML = "";
4)
document.getElementById("inputForm").parentElement.removeChile(document.getElementById("inputForm"));
innerHTMLプロパティ
以下は innerHTML = 値 としている
値が上書きされるので、最後のquxしか入らない
<ul id="list"></ul>
var ulElement = document.getElementById('list');
ulElement.innerHTML = '<li>foo</li>';
ulElement.innerHTML = '<li>baz</li>';
ulElement.innerHTML = '<li>qux</li>';
+= と書けば値が全て追加される
<ul id="list"></ul>
var ulElement = document.getElementById('list');
ulElement.innerHTML += '<li>foo</li>';
ulElement.innerHTML += '<li>baz</li>';
ulElement.innerHTML += '<li>qux</li>';
この記事が気に入ったらサポートをしてみませんか?