『四科のまとめ』算数Pythonコード
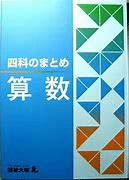
def calculate_amounts(compare_amount=None, base_amount=None, ratio=None):
"""
くらべる量、もとにする量、割合のいずれか2つが与えられたときに残りの1つを計算します。
"""
if compare_amount is None and base_amount is not None and ratio is not None:
# くらべる量 = もとにする量 × 割合
compare_amount = base_amount * ratio
elif base_amount is None and compare_amount is not None and ratio is not None:
# もとにする量 = くらべる量 ÷ 割合
base_amount = compare_amount / ratio
elif ratio is None and compare_amount is not None and base_amount is not None:
# 割合 = くらべる量 ÷ もとにする量
ratio = compare_amount / base_amount
return compare_amount, base_amount, ratio
# 与えられた値(例: 食塩、食塩水、濃さ)
compare_amount = None # くらべる量
base_amount = 200 # もとにする量 (食塩水の量)
ratio = 0.05 # 割合 (濃さ)
# 計算
result_compare_amount, result_base_amount, result_ratio = calculate_amounts(compare_amount, base_amount, ratio)
# 結果を表示
print(f"くらべる量: {result_compare_amount}g" if result_compare_amount is not None else "くらべる量は計算できません")
print(f"もとにする量: {result_base_amount}g" if result_base_amount is not None else "もとにする量は計算できません")
print(f"割合: {result_ratio}" if result_ratio is not None else "割合は計算できません")
def calculate_salt_concentration(salt=None, solution=None, concentration=None):
"""
食塩、食塩水、濃さのいずれか2つの値が与えられたときに残りの1つを計算します。
"""
if salt is None and solution is not None and concentration is not None:
# 食塩が与えられていないとき、食塩 = 食塩水 × 濃さ
salt = solution * concentration
elif solution is None and salt is not None and concentration is not None:
# 食塩水が与えられていないとき、食塩水 = 食塩 ÷ 濃さ
solution = salt / concentration
elif concentration is None and salt is not None and solution is not None:
# 濃さが与えられていないとき、濃さ = 食塩 ÷ 食塩水
concentration = salt / solution
return salt, solution, concentration
# 与えられた値
salt = 10 # 食塩の量 (g)
solution = 200 # 食塩水の量 (g)
concentration = None # 濃さ(割合)
# 計算
result_salt, result_solution, result_concentration = calculate_salt_concentration(salt, solution, concentration)
# 結果を表示
print(f"食塩: {result_salt}g" if result_salt is not None else "食塩は計算できません")
print(f"食塩水: {result_solution}g" if result_solution is not None else "食塩水は計算できません")
print(f"濃さ: {result_concentration}" if result_concentration is not None else "濃さは計算できません")
import matplotlib.pyplot as plt
import numpy as np
def calculate_polygon_properties(N):
"""
Calculate the sum of interior angles and the number of diagonals for an N-sided polygon.
Parameters:
N (int): The number of sides of the polygon
Returns:
tuple: Sum of interior angles, Number of diagonals
"""
if N < 3:
raise ValueError("N must be 3 or greater for a valid polygon.")
# Calculate the sum of interior angles
interior_angle_sum = 180 * (N - 2)
# Calculate the number of diagonals
diagonals_count = (N * (N - 3)) // 2
return interior_angle_sum, diagonals_count
# Set the range of N
N_values = np.arange(3, 21) # From 3-sided polygon to 20-sided polygon
# Calculate the sums of interior angles and the number of diagonals
interior_angles = [calculate_polygon_properties(N)[0] for N in N_values]
diagonals = [calculate_polygon_properties(N)[1] for N in N_values]
# Create the plots
plt.figure(figsize=(12, 6))
# Plot for the sum of interior angles
plt.subplot(1, 2, 1)
plt.plot(N_values, interior_angles, marker='o', color='blue')
plt.title('Sum of Interior Angles of N-sided Polygon')
plt.xlabel('Number of Sides (N)')
plt.ylabel('Sum of Interior Angles (degrees)')
plt.grid(True)
# Plot for the number of diagonals
plt.subplot(1, 2, 2)
plt.plot(N_values, diagonals, marker='o', color='red')
plt.title('Number of Diagonals in N-sided Polygon')
plt.xlabel('Number of Sides (N)')
plt.ylabel('Number of Diagonals')
plt.grid(True)
# Display the plots
plt.tight_layout()
plt.show()
import math
def calculate_sector_properties(radius, angle_degrees):
"""
Calculate the arc length and area of a sector given the radius and central angle in degrees.
Parameters:
radius (float): The radius of the sector
angle_degrees (float): The central angle of the sector in degrees
Returns:
tuple: Arc length, Area of the sector
"""
# Convert angle from degrees to radians for calculation
angle_radians = math.radians(angle_degrees)
# Calculate the arc length
arc_length = radius * angle_radians
# Calculate the area
area = 0.5 * radius**2 * angle_radians
return arc_length, area
# Example values
radius = 5 # Radius of the sector
angle_degrees = 60 # Central angle in degrees
# Calculate
arc_length, area = calculate_sector_properties(radius, angle_degrees)
# Display results
print(f"Arc Length: {arc_length:.2f}")
print(f"Area: {area:.2f}")
import numpy as np
import matplotlib.pyplot as plt
# Define the coefficients matrix A and the constants vector B
A = np.array([
[2, 3], # Coefficients for the first equation
[4, -1] # Coefficients for the second equation
])
B = np.array([
[5], # Right-hand side of the first equation
[1] # Right-hand side of the second equation
])
# Solve the system of equations
solution = np.linalg.solve(A, B)
# Print the solution
print("The solution is:")
print(f"x = {solution[0][0]}")
print(f"y = {solution[1][0]}")
# Plotting
x = np.linspace(-5, 5, 400)
# Equations of the lines
y1 = (5 - 2*x) / 3
y2 = (4*x - 1) / 1
plt.figure(figsize=(8, 6))
# Plot the lines
plt.plot(x, y1, label='2x + 3y = 5', color='blue')
plt.plot(x, y2, label='4x - y = 1', color='red')
# Plot the solution point
plt.plot(solution[0][0], solution[1][0], 'go', label=f'Solution ({solution[0][0]:.2f}, {solution[1][0]:.2f})')
# Labels and title
plt.xlabel('x')
plt.ylabel('y')
plt.title('Graph of the Linear Equations')
plt.axhline(0, color='black',linewidth=0.5)
plt.axvline(0, color='black',linewidth=0.5)
plt.grid(color = 'gray', linestyle = '--', linewidth = 0.5)
plt.legend()
# Show plot
plt.show()
def volume_conversion(dL=None, mL=None, L=None):
if dL is not None:
mL_value = dL * 100
L_value = dL / 10
return mL_value, L_value
elif mL is not None:
dL_value = mL / 100
L_value = mL / 1000
return dL_value, L_value
elif L is not None:
dL_value = L * 10
mL_value = L * 1000
return dL_value, mL_value
else:
return None, None
def area_conversion(km2=None, ha=None, a=None):
if km2 is not None:
m2_value = km2 * 1_000_000
ha_value = km2 * 100
a_value = km2 * 10_000
return m2_value, ha_value, a_value
elif ha is not None:
m2_value = ha * 10_000
km2_value = ha / 100
a_value = ha * 100
return m2_value, km2_value, a_value
elif a is not None:
m2_value = a * 100
km2_value = a / 10_000_000
ha_value = a / 100
return m2_value, km2_value, ha_value
else:
return None, None, None
# Example conversions
print("Volume Conversions:")
dL_to_mL, dL_to_L = volume_conversion(dL=2)
print(f"2 dL = {dL_to_mL} mL")
print(f"2 dL = {dL_to_L} L")
mL_to_dL, mL_to_L = volume_conversion(mL=200)
print(f"200 mL = {mL_to_dL} dL")
print(f"200 mL = {mL_to_L} L")
L_to_dL, L_to_mL = volume_conversion(L=5)
print(f"5 L = {L_to_dL} dL")
print(f"5 L = {L_to_mL} mL")
print("\nArea Conversions:")
km2_to_m2, km2_to_ha, km2_to_a = area_conversion(km2=3)
print(f"3 km² = {km2_to_m2} m²")
print(f"3 km² = {km2_to_ha} ha")
print(f"3 km² = {km2_to_a} a")
ha_to_m2, ha_to_km2, ha_to_a = area_conversion(ha=7)
print(f"7 ha = {ha_to_m2} m²")
print(f"7 ha = {ha_to_km2} km²")
print(f"7 ha = {ha_to_a} a")
a_to_m2, a_to_km2, a_to_ha = area_conversion(a=50)
print(f"50 a = {a_to_m2} m²")
print(f"50 a = {a_to_km2} km²")
print(f"50 a = {a_to_ha} ha")
def calculate_salt_concentration(salt=None, solution=None, concentration=None):
"""
Given two of salt, solution, or concentration, calculate the remaining one.
"""
if salt is None and solution is not None and concentration is not None:
# When salt is not provided, calculate salt = solution × concentration
salt = solution * concentration
elif solution is None and salt is not None and concentration is not None:
# When solution is not provided, calculate solution = salt ÷ concentration
solution = salt / concentration
elif concentration is None and salt is not None and solution is not None:
# When concentration is not provided, calculate concentration = salt ÷ solution
concentration = salt / solution
return salt, solution, concentration
def calculate_distance(speed, time):
"""Calculate distance given speed and time."""
if speed is None or time is None:
raise ValueError("Speed and time must not be None for distance calculation.")
return speed * time
def calculate_time(distance, speed):
"""Calculate time given distance and speed."""
if distance is None or speed is None:
raise ValueError("Distance and speed must not be None for time calculation.")
if speed == 0:
raise ValueError("Speed cannot be zero for time calculation.")
return distance / speed
def calculate_speed(distance, time):
"""Calculate speed given distance and time."""
if distance is None or time is None:
raise ValueError("Distance and time must not be None for speed calculation.")
if time == 0:
raise ValueError("Time cannot be zero for speed calculation.")
return distance / time
# Example for salt concentration
salt = 10 # grams of salt
solution = 200 # grams of solution
concentration = None # concentration (fraction)
# Calculate salt concentration
result_salt, result_solution, result_concentration = calculate_salt_concentration(salt, solution, concentration)
# Display results for salt concentration
print(f"Salt: {result_salt}g" if result_salt is not None else "Salt cannot be calculated")
print(f"Solution: {result_solution}g" if result_solution is not None else "Solution cannot be calculated")
print(f"Concentration: {result_concentration}" if result_concentration is not None else "Concentration cannot be calculated")
# Example for distance-speed-time calculations
speed = 60 # km/h
time = 2 # hours
distance = None # km
# Calculate distance
if speed is not None and time is not None:
calculated_distance = calculate_distance(speed, time)
print(f"Distance = Speed × Time: {speed} km/h × {time} hours = {calculated_distance} km")
else:
print("Speed or time is None, cannot calculate distance.")
# Calculate time
if distance is not None and speed is not None:
calculated_time = calculate_time(distance, speed)
print(f"Time = Distance ÷ Speed: {distance} km ÷ {speed} km/h = {calculated_time} hours")
else:
print("Distance or speed is None, cannot calculate time.")
# Calculate speed
if distance is not None and time is not None:
calculated_speed = calculate_speed(distance, time)
print(f"Speed = Distance ÷ Time: {distance} km ÷ {time} hours = {calculated_speed} km/h")
else:
print("Distance or time is None, cannot calculate speed.")
import math
# Define the radius
radius = 10
# Define the value of π
pi = 3.14
# Calculate the area of a quarter circle
quarter_circle_area = (pi * radius**2) / 4
# Calculate the area of a right isosceles triangle
triangle_area = (radius**2) / 2
# Calculate the area of a segment (chord area)
chord_area = quarter_circle_area - triangle_area
# Calculate the area of the leaf shape (2 segments)
leaf_shape_area = chord_area * 2
print(f"Area of the leaf shape: {leaf_shape_area} cm^2")
# For the second problem with a radius of 20
radius = 20
# Calculate the area of a quarter circle
quarter_circle_area = (pi * radius**2) / 4
# Calculate the area of a right isosceles triangle
triangle_area = (radius**2) / 2
# Calculate the area of a segment (chord area)
chord_area = quarter_circle_area - triangle_area
# Calculate the area for 3 quarter circles
three_ovals_area = chord_area * 3
print(f"Area of the three quarter circles: {three_ovals_area} cm^2")
# 数列AのN番目の値を求める関数
def nth_term_of_A(n):
# Aの最初の値
a1 = 2
# Bのn-1番目までの和を求める
sum_of_B = (1 + (n - 1)) * (n - 1) // 2
# Aのn番目の値
return a1 + sum_of_B
# Aの値が80になるのが何番目かを求める関数
def find_position_in_A(target_value):
n = 1
while True:
a_n = nth_term_of_A(n)
if a_n == target_value:
return n
elif a_n > target_value:
return -1 # もし見つからない場合は-1を返す
n += 1
# Aの10番目の値を求める
a_10 = nth_term_of_A(10)
print(f"Aの10番目の値は: {a_10}")
# Aの値が80になるのが何番目かを求める
position_of_80 = find_position_in_A(80)
if position_of_80 != -1:
print(f"80はAの{position_of_80}番目の値です。")
else:
print("80はAに存在しません。")
import numpy as np
# 等差数列の問題
def arithmetic_sequence_example():
a1 = 1 # 初項
d = 4 # 公差
n1 = 17 # 17番目の項を求める
n2 = 10 # 1番目から10番目までの和を求める
# n番目の項を求める公式
an1 = a1 + (n1 - 1) * d
print(f"等差数列の17番目の項: {an1}")
# 和の公式
S_n2 = n2 * (a1 + (a1 + (n2 - 1) * d)) / 2
print(f"等差数列の1番目から10番目までの和: {S_n2}")
# 等比数列の問題
def geometric_sequence_example():
a1 = 1 # 初項
r = 2 # 公比
n = 8 # 128までの和を求める(8項目)
# 和の公式
S_n = a1 * (r**n - 1) / (r - 1)
print(f"等比数列の1から128までの和: {S_n}")
# 練習問題2
a1 = 2 # 初項
r = 3 # 公比
n = 6 # 1458までの和を求める(6項目)
# 和の公式
S_n2 = a1 * (r**n - 1) / (r - 1)
print(f"等比数列の1から1458までの和: {S_n2}")
# 階差数列の問題
def difference_sequence_example():
a1 = 1 # 初項
n = 10 # 10番目の項を求める
# 各項の差が等差数列
sum_diff = sum(range(1, n)) # 1 + 2 + 3 + ... + 9
an = a1 + sum_diff
print(f"階差数列の10番目の項: {an}")
# 実行
arithmetic_sequence_example()
geometric_sequence_example()
difference_sequence_example()
def tsuru_kame(heads, legs):
"""ツルとカメの数を計算する"""
tsuru_count = (heads * 4 - legs) // 2
kame_count = heads - tsuru_count
return tsuru_count, kame_count
def meeting_time(distance, speed1, speed2):
"""二人が出会う時間を計算する"""
return distance / (speed1 + speed2)
def catching_up_time(distance, speed1, speed2):
"""後ろの人が追いつく時間を計算する"""
return distance / abs(speed1 - speed2)
# ツルカメ算の例
heads = 10
legs = 28
tsuru_count, kame_count = tsuru_kame(heads, legs)
print(f"ツルの数: {tsuru_count}")
print(f"カメの数: {kame_count}")
# 旅人算の例1: 二人が出会う時間
distance_meeting = 60
speed1_meeting = 10
speed2_meeting = 15
meeting = meeting_time(distance_meeting, speed1_meeting, speed2_meeting)
print(f"出会う時間: {meeting:.2f} 時間")
# 旅人算の例2: 後ろの人が追いつく時間
distance_catching = 50
speed1_catching = 12
speed2_catching = 8
catching_up = catching_up_time(distance_catching, speed1_catching, speed2_catching)
print(f"追いつく時間: {catching_up:.2f} 時間")
# 旅人算の公式 (Traveler's Problem Formulas)
def meeting_time(distance, speed1, speed2):
"""2人が反対方向に進んで出会う時間を求める (Find the time until two people meet moving in opposite directions)"""
return distance / (speed1 + speed2)
def catching_up_time(initial_distance, speed1, speed2):
"""同じ方向に進んで後ろの人が前の人に追いつく時間を求める (Find the time until a person catches up with another moving in the same direction)"""
return initial_distance / abs(speed1 - speed2)
# つるかめ算の公式 (Crane and Turtle Problem Formulas)
def turtles_count(total_heads, total_legs):
"""カメの数を求める (Find the number of turtles given the total number of heads and legs)"""
return (total_legs - 4 * total_heads) // -2
def cranes_count(total_heads, total_legs):
"""ツルの数を求める (Find the number of cranes given the total number of heads and legs)"""
return (4 * total_heads - total_legs) // 2
# 植木算の公式 (Tree Planting Problem Formulas)
def trees_in_line_with_ends(distance_between_trees, trees_with_ends):
"""一直線上に木が立っていて、両端にも木がある場合の木の数 (Find the number of trees in a line with trees at both ends)"""
return distance_between_trees + 1
def trees_in_line_without_ends(distance_between_trees, trees_without_ends):
"""一直線上に木が立っていて、両端にも木がない場合の木の数 (Find the number of trees in a line with no trees at the ends)"""
return distance_between_trees - 1
def trees_in_circle(distance_between_trees, trees_in_circle):
"""円形に木を植える場合の木の数 (Find the number of trees planted in a circle)"""
return distance_between_trees
# 通過算の公式 (Passing Problem Formulas)
def overtaking_time(length_train1, length_train2, speed1, speed2):
"""追い越すときの時間を求める (Find the time to overtake another train)"""
return (length_train1 + length_train2) / abs(speed1 - speed2)
def crossing_time(length_train1, length_train2, speed1, speed2):
"""すれ違うときの時間を求める (Find the time to pass by another train)"""
return (length_train1 + length_train2) / (speed1 + speed2)
# 使用例 (Usage Examples)
print("出会うまでの時間:", meeting_time(100, 60, 40)) # 100kmの距離で、速さが60km/hと40km/hの場合 (Time to meet with 100 km distance, speeds of 60 km/h and 40 km/h)
print("追いつくまでの時間:", catching_up_time(50, 60, 40)) # 初期距離が50kmで、速さが60km/hと40km/hの場合 (Time to catch up with initial distance of 50 km, speeds of 60 km/h and 40 km/h)
print("カメの数:", turtles_count(10, 28)) # 頭数10で足の合計が28 (Number of turtles with 10 heads and 28 legs)
print("ツルの数:", cranes_count(10, 28)) # 頭数10で足の合計が28 (Number of cranes with 10 heads and 28 legs)
print("両端にも木がある場合の木の数:", trees_in_line_with_ends(5, 6)) # 木と木の間が5mで、両端に木がある (Number of trees in a line with trees at both ends and 5 meters between them)
print("両端に木がない場合の木の数:", trees_in_line_without_ends(5, 4)) # 木と木の間が5mで、両端に木がない (Number of trees in a line with no trees at the ends and 5 meters between them)
print("円形に木を植える場合の木の数:", trees_in_circle(8, 8)) # 木と木の間が8mで、円形に植える場合 (Number of trees planted in a circle with 8 meters between them)
print("追い越すときの時間:", overtaking_time(100, 120, 80, 60)) # 列車1の長さ100m、列車2の長さ120m、速さが80km/hと60km/hの場合 (Time to overtake with train lengths of 100 m and 120 m, speeds of 80 km/h and 60 km/h)
print("すれ違うときの時間:", crossing_time(100, 120, 80, 60)) # 列車1の長さ100m、列車2の長さ120m、速さが80km/hと60km/hの場合 (Time to cross with train lengths of 100 m and 120 m, speeds of 80 km/h and 60 km/h)
この記事が気に入ったらサポートをしてみませんか?